For the second assignment, I took inspiration from Random Squares by Bill Solomyjec.
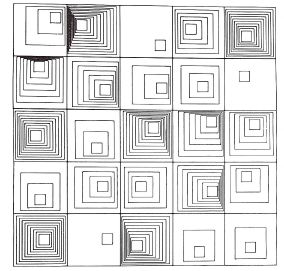
I liked the idea of incorporating randomness in a controlled manner and how each square was unique.
Implementation
I figured that I needed to first created a grid within my canvas. I was able to do this by using variables and loops to divide the canvas evenly.
let square_length = 80;
let num_rows = 5;
let num_cols = 8
createCanvas(square_length*num_cols, square_length*num_rows);
for(let row = 0; row <num_rows;row+=1){
for(let col = 0; col<num_cols;col+=1){
let startx = col*square_length;
let starty = row*square_length;
fill(255)
square(startx,starty,square_length)
}
}
Then I figured for each square within the grid, I needed a number of random values: an offset for the initial square, a random ratio in the x and y direction for the squares to grow, and a random gap between each square to multiply the ratios by.
I implemented them via multiple 2D arrays in the following way:
for(let row = 0; row <num_rows;row+=1){
currrow = []
for(let col = 0; col<num_cols;col+=1){
currrow.push([random(minoffset,square_length-base_length-1),random(minoffset,square_length-base_length-1)])
}
offsetlist.push(currrow);
};
for(let row = 0; row <num_rows;row+=1){
currrow = []
for(let col = 0; col<num_cols;col+=1){
currrow.push([random(maxratio),random(maxratio)]);
}
ratiolist.push(currrow);
};
for(let row = 0; row <num_rows;row+=1){
currrow = []
for(let col = 0; col<num_cols;col+=1){
currrow.push(random(mingap,maxgap));
}
gaplist.push(currrow);
};
Then, using these values, I could draw each square in each grid location by extracting these random values as I drew each grid square.
for(let row = 0; row <num_rows;row+=1){
for(let col = 0; col<num_cols;col+=1){let [xoffset, yoffset] = offsetlist[row][col]
let [xratio, yratio] = ratiolist[row][col]
let squaregap = gaplist[row][col]
for(let sqoffset=0; sqoffset<min(xoffset/xratio,yoffset/yratio); sqoffset+=squaregap){
let offsetedx = startx+xoffset-sqoffset*xratio;
let offsetedy = starty+yoffset-sqoffset*yratio;
let offsetedlength = base_length+sqoffset*(1+max(xratio,yratio))
square(offsetedx,offsetedy,offsetedlength);
}
}
}
The min(xoffset/xratio,yoffset/yratio) in the innermost for loop controls the boundaries of the largest square, ensuring that the squares do not extend beyond the top or left of the grid. This prevents a lot of the potential chaos.