For the midterm, I wanted to work on a game that was in the board games genre of games, and thus, I decided to take a spin on Snakes and Ladders. Snakes and Ladders is a board game with a grid of numbers. It is a multi-player game and the target is to reach the 100th block from the 1st block. The movement of the tokens is decided by a die and if you happened to land on a square with a snake or a ladder – you’ll either move down a lot of squares or move up respectively.
Setting the elements of the game
On brainstorming how I could rethink this game, I ended up deciding on having the elements of cards in the game. In addition, I started off with a lot of variations for the pathway: from spirals to a boat path to circles – but I finalized on a grid-style format so that, the familiarity of the grid-style game plays into easing the player into learning how to play the game.
Instead of the elements of snakes and ladders, I put in cards on random squares on the grid – these squares are randomly picked at the beginning of every game – on landing on the square – the player would have to look at the corresponding card and do what the instructions say (related to moving on the squares). These cards are related to my home in Kerala and things that a person from Kerala, India would be familiar with. I wanted to make the cards accessible for anyone, without context to understand – so, there will be text included that will dictate the movement of the user token. Types of cards would include:
-
-
- Move-ahead cards: Examples – Dosa, Grandma’s Food, Filter Coffee, etc.
- Move-behind cards: Examples – Powercuts, Humungous mosquitoes, Coconut falling on your head, etc.
-
I tried making these cards using images I could find online but since the styles of art depicting all of these ideas were different, it looked out of place. So, I decided to draw the card art on my own for the game – to keep the aesthetics consistent.
Finally, since it is a multiplayer game – I decided to limit the number to two people to play the game. But if I have the time, I might consider improving the game by having the players input the number of players. For now, the user tokens are images from celebrated artforms in Kerala – Kathakali & Theyyam. Depending on whose turn it is, the corresponding token lights up and the other token is grayed out.
Implementation
I started off working on the grid. The grid is made by putting together a number of squares – so I made a class called Rect and a function called grid() which was responsible for making the objects of class Rect and numbering and displaying them. Having classes here helped a lot in adding additional functionality like putting in the number of the rectangle when displaying. For the later part of implementation too, this would greatly help in terms of randomly assigning squares in the grid and have them light a different color to represent the snake or ladder element.
//grid print void grid(){ int xlen = (width)/size ; int ylen = (height)/size; rect = new Rect[(xlen-7)*(ylen)]; int i=0; for (int y=0; y < ylen; y++) { for (int x = 3; x <xlen-4; x++) { PVector p = new PVector(x*size,y*size); rect[i] = new Rect(p, size,i); i++; } } } class Rect{ float size; PVector position; int num; Rect(PVector p, float s, int n){ position = p; size = s; num=n; } void display(){ stroke(255); fill(0); rect(position.x,position.y,size,size); fill(255); text(num,position.x,position.y+size-5); } }
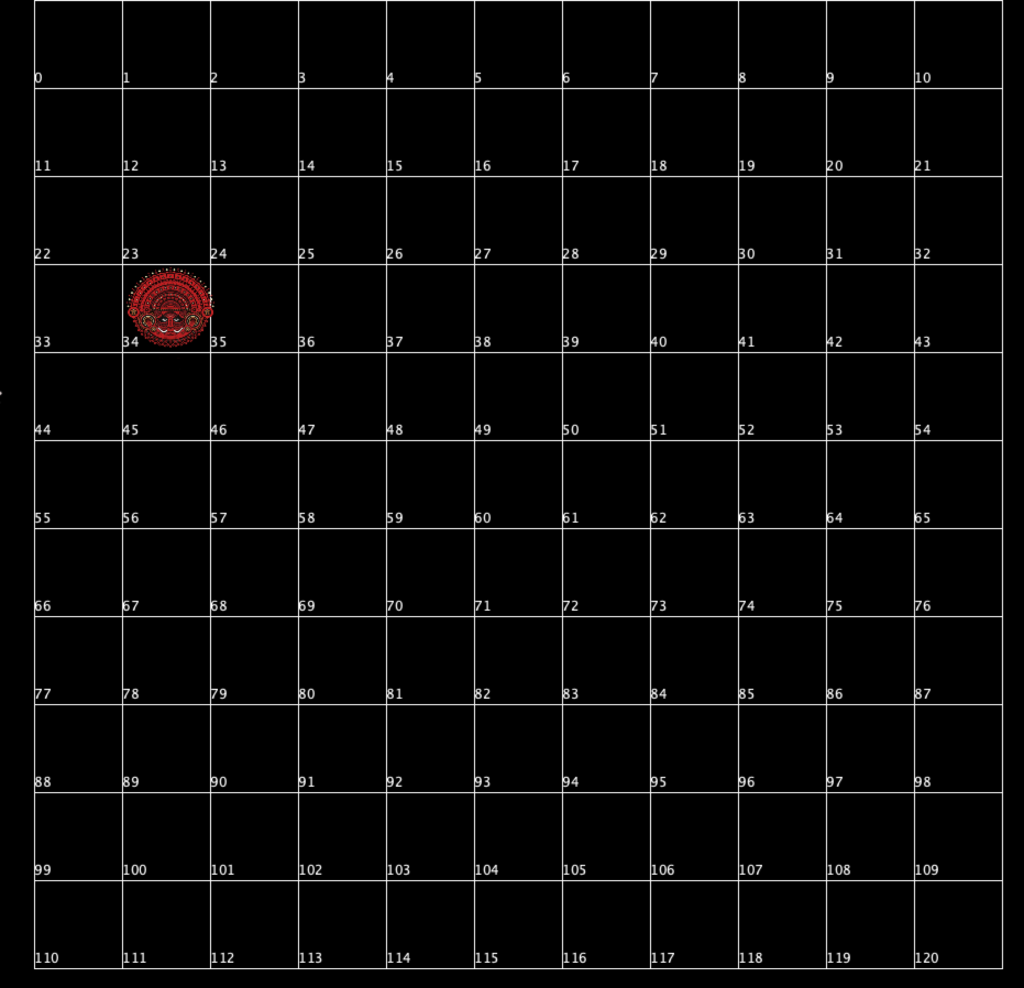
Then, I worked on implementing a die program on a separate processing sketch. I used this link as a reference to work on this. When you press within the die, it stops otherwise it keeps rolling.
void dice(){ fill(255); text("Click on the dice to roll it, Player 1", diceX-diceSize,diceY-diceSize+20); fill(#FFF3D6); rectMode(CENTER); rect(diceX, diceY, diceSize, diceSize, diceSize/5); //dots fill(50); int side = int(random(1, 7)); if (side == 1 || side == 3 || side == 5) ellipse(diceX, diceY, diceSize/5, diceSize/5); if (side == 2 || side == 3 || side == 4 || side == 5 || side == 6) { ellipse(diceX - diceSize/4, diceY - diceSize/4, diceSize/5, diceSize/5); ellipse(diceX+ diceSize/4, diceY + diceSize/4, diceSize/5, diceSize/5); } if (side == 4 || side == 5 || side == 6) { ellipse(diceX - diceSize/4,diceY + diceSize/4, diceSize/5, diceSize/5); ellipse(diceX + diceSize/4, diceY- diceSize/4, diceSize/5, diceSize/5); } if (side == 6) { ellipse(diceX, diceY- diceSize/4, diceSize/5, diceSize/5); ellipse(diceX, diceY + diceSize/4, diceSize/5, diceSize/5); } rectMode(CORNER); } void mousePressed(){ if(mouseX>(diceX)-(diceSize/2) && mouseX<(diceX)+(diceSize/2) && mouseY>(diceY)-(diceSize/2) && mouseY<(diceY)+(diceSize/2 )) toggleRun=!toggleRun; }
I combined all of these together and finally, put in the user tokens on the side indicating whose turn it is.
PImage kathakali,kathakaliInactive; PImage theyyam,theyyamInactive; Rect rect[]; int size = 80; int diceX = (100*width/8)+25; int diceY =(60*height/8)+10; int diceSize = 90; boolean toggleRun=false; void setup() { fullScreen(); background(0); theyyam = loadImage("theyyam.png"); theyyamInactive = loadImage("theyyamInactive.png"); theyyam.resize(220, 260); theyyamInactive.resize(220, 260); kathakali = loadImage("kathakali.png"); kathakaliInactive = loadImage("kathakaliInactive.png"); kathakali.resize(180,224); kathakaliInactive.resize(180,224); //how to play //players choose whether they want theyyam or kathakali image(theyyamInactive, 0, height/2-190); image(kathakaliInactive, 15, height/2+20); dice(); } void draw() { grid(); for (int j=0; j<rect.length; j++){ rect[j].display(); } scale(0.4); image(theyyam, width/2+80, height/2+140); if(toggleRun){ for(int i=0;i<10;i++) { dice(); } } }
Next Steps:
-
- Add in a beginning and final sketch for the game
- Add an instructions page
- Random function to assign cards to squares and change their colors
- Make the movement function, so that the tokens move according to the die input and the cards, if applicable.
- Celebration visuals when one of the players wins – My idea is to have the grid light up in all sorts of colors to generate excitement.
References:
Looks great Gopika! You drew those?? Looking forward to seeing how it develops.
Thank you professor! Just realised my reply didn’t get posted, but those works were done by other artists, will make sure to credit them in my final documentation!