This week was an especially difficult one because I had a lot of deadlines and tasks and responsibilities that needed to be done within the short time period of a week and adding to that I was just finding it quite hard to get the inspiration to create a piece of art. But thankfully it finally dawned on me and I decided to pursue a rather cliche idea but give it a twist thanks to all that I have learned in the recent days about Processing.
So I ended up creating my own idea of the big bang and created a star that can be controlled to be bigger or smaller and if it is bigger than a specific size, it erupts and ends up in an asteroid blast.
Difficulties:
- As I mentioned before, I was really struggling to get an idea this week and tried coming up with multiple ideas to see what might work. However, none of them seem to really appeal to me but thankfully I decided to pursue the Big Bang Idea in the end.
- The big challenge that I was facing apart from actually getting an idea to make something, was to get the asteroids to work. I had experimented with multiple different ways of trying to make them appear out of the screen but every time I tried a technique I would run into some kind of problems. Sometimes, the asteroids would not spawn properly, other times they would not move the way I wanted them to (appear out of a screen in a 3D sort of fashion) and basically would not give out the right sense of an asteroid blast that I had imagined.
Resolution:
- In order to solve the asteroid problem, I decided to watch some videos that TheCodingTrain had made regarding a similar problem and found out that the best solution to this is to actually use the concept of classes. And so to implement that I made an asteroid class and added the functions, show and update to it. This allowed me to create multiple asteroids and then manipulate them in the way I wanted. Thus, the utilization of OOP helped me solve this problem.
Elements in the project
- The project has two scenes – one of an exploding star and the other that of an asteroid blast. It revolves around the concept of a big star bursting so as to create an asteroid shower. The player can control the size of the star – making it bigger or smaller. Once the star reaches a certain limit of the size, going beyond that would cause it to explode and release an asteroid shower. At every point in the visual, the user can pause the animation and play it again and can also make it go faster or slower (done by altering the Frame Rate). During the asteroid shower, the user can control the speed by which the asteroids come using the mouse. Sliding it to the right will increase the speed whereas going to the left will decrease it.
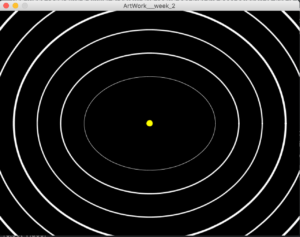
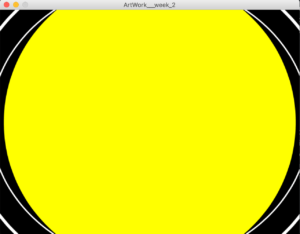
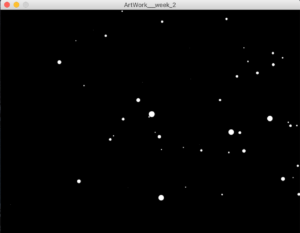
Concepts Learned:
- This project taught me how to better utilize the random and noise functions better along with obviously teaching me how to use loops to create art works. By using random and noise in this project, I sort of got this understanding that a noise is a more controlled expression of generating a random number between a specified range and the fact that its value is retained (or remembered) whereas in random I feel like the generation of random numbers is more uncontrolled and more dispersed if I were to say.
- I learned to implement the concepts of OOP in processing.
Project Video:
I will be uploading a vlog about my experience with this project soon by tonight!
Project Code:
//this artwork is a depiction of a big bang and the asteroids that come after that float speed = 0.01; //for noise value of the star, determines the pace of the vibration like movement float extent = 0.7; //for noise value of the star, determines the limit to which size comes up and goes down int StarSize = 10; // variable star size that increases and decreases float StrokeSpeed = .01; float StrokeExtent = 0.7; int StrokeSize = 5; //for the throbbing effect of orbits float noiseStrokeValue; float noiseStarValue; color bg_color; float Asteroid_speed; //to determine speed of asteroid class Asteroid { float Asteroid_x; float Asteroid_y; float Asteroid_d; Asteroid() { Asteroid_x = random(-width, width); Asteroid_y = random(-height, height); Asteroid_d = random(width); //helps create an illusion that it is a 3d space } void update() { Asteroid_d -= Asteroid_speed; if (Asteroid_d < 1) { Asteroid_x = random(-width, width); Asteroid_y = random(-height, height); Asteroid_d = width; } } //allows for movement void show_Asteroid() { fill(255); noStroke(); float sx = map(Asteroid_x/Asteroid_d, 0, 1, 0, width); float sy = map(Asteroid_y/Asteroid_d, 0, 1, 0, height); //taking a ratio and then increasing and decreasing it to make it look 3d float size = map(Asteroid_d, 0, width, 16, 0); ellipse(sx, sy, size, size); } //shows random asteroids on screen }; //Asteroid class to create asteroids and to update and show them on screen Asteroid[] asteroids = new Asteroid[400]; // 400 Asteroids created void setup() { size(640, 480); for (int i = 0; i<asteroids.length; i++) { asteroids[i] = new Asteroid(); // each element is made into an instance of the Asteroid class } } void draw() { background(bg_color); Asteroid_speed = map(mouseX, 0, width, 0, 20); //speed controlled by mouse Pointer if (StarSize < 510) //first star gets bigger Big_Bang(); else AfterMath(); //after a certain size it bursts and asteroids follow } void Big_Bang() { bg_color = color(0); noFill(); translate(width/2, height/2); stroke(255); noFill(); for (int s=2; s<8; s++) { strokeWeight(random(s)); arc(0, 0, s*100+80, s*100, 0, TWO_PI); //6 orbits created } noiseStrokeValue = noise(frameCount*StrokeSpeed); noiseStrokeValue += StrokeExtent; noiseStrokeValue *= StrokeSize; //adding flashing effect to orbit noiseStarValue = noise(frameCount*speed); noiseStarValue += extent; noiseStarValue *= StarSize; //adding movement to star stroke(255, 255, 0); fill(255, 255, 0); strokeWeight(noiseStrokeValue); circle(0, 0, noiseStarValue); //making star } void AfterMath() { translate(width/2, height/2); for (int i = 0; i<asteroids.length; i++) { asteroids[i].update(); asteroids[i].show_Asteroid(); } } void keyPressed() { if (key == 'a') //slow { frameRate(frameRate-20); } if (key == 'b') //pause { noLoop(); } if (key == 'c') //play { loop(); } if (key == 'k') //fast { frameRate(frameRate+20); } if (key == 'w') //increase_Star_size { StarSize += 50; print(StarSize); } if (key == 's') //decrease_Star_size { StarSize -= 50; print(StarSize); } } void keyReleased() { frameRate(60); }