Intro + Idea
This weekend, I visited an art exhibition in a space called Warehouse 421 in Mina Zayed. It was my first time exploring a creative space other than the Louvre, and I realized that I wanted to make a checklist of some of the main places that I would like to visit during my time here. Something else I realized, is that sometimes it’s hard to visualize how far something is in the UAE (does that make sense?), I’m still constructing a mental map of Abu Dhabi but find myself still needing to refer to Google Maps to make an estimate of the distance.
This inspired my game, I wanted to show the user pictures of some art/creative spaces in the UAE, and for them to estimate how far they are by interacting with the sensor.
Initial Attempts
Again, I wanted to experiment with the Ultrasonic Sensor. I spent a lot of time understanding how it works, and the conversions needed to change time to distance. I found it really fascinating and actually recalled that it isn’t my first experience with the sensor! I used to use it in robotics back in high school, but I guess I didn’t have that clear of an understanding of it.
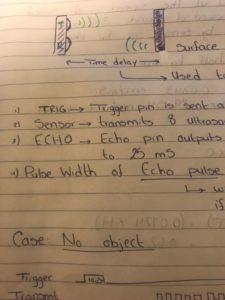
This video was really helpful in terms of understanding the functionality and conversion. However, I struggled a lot with the serial communication part. I was initially able to print out the distance in CM on the screen but then was faced with a lot of difficulties and errors when it came to working with the value for the game functionality.
Final Idea
So, for this one, I switched to the light sensor/photocell and thought it could be interesting to see how one could map brightness to distance. I completed my communication using the ascii handshake, as I wanted the full range of readings.
For the game, I created an array of locations in the UAE and another array of distances (referring to the distance from campus). I created three categories:
- 1-10 KM Away
- 11 – 20 KM Away
- Outside the City
Then, based on the distance array I classified all the locations in the group. As for the input, I created brightness ranges, i.e. if the brightness was between 50- 200 (sensor covered), the spot is in the 1 – 10 KM category…etc. Whatever the user inputs is then saved in a variable that is used to compare with the “correctCategory”. So, if the user gets “Louvre Abu Dhabi”, they’re meant to cover the sensor. If they do, their input value saves UserInputCategory as 1, and hence it shows a correct message when it is compared to the CorrectCategory variable.
I tried to make the sequences logical in terms of cognitive mapping and how the user interacts with the game, rather than ordered in the code. Meaning that category 1 has the darkest range while category 2 has the brightest, and 3 (actually farthest from the sensor and farthest from campus) has a brightness in between.
Here’s an example of the gameplay, I’m missing a start screen and a replay button since I focused on getting this part to work. As you can see, I had indicators on my desk, the first one indicating spaces that are within the city but a bit farther from campus, and the second one indicating spaces outside of the city. So, for the second example, when I got “Alserkal Avenue” located in Dubai, it only worked when I moved to the suitable indicator.
However, this is not always the case, there’s a glitch in the code, and I’m afraid it might be how sensitive the photocell is, as sometimes it shows the “correct” message at the wrong time.
Possible Developments
I found myself understanding the concepts of serial communication we went over in class, and the other examples but struggled with the application a bit, especially with the distance sensor. So trying to work with it more could be helpful before starting to work on the final project.
A nice development/addition to this game would be inquiring about estimate cab price rather than distance. I found that many students estimate how far someplace is by how much a cab would cast. e.g. 50 AED to YAS mall, so anything around that would cost around the same amount. It might be more relatable and interesting that way!
Arduino Code:
int photoCell = A0; void setup() { // put your setup code here, to run once: Serial.begin(9600); Serial.println('0'); //initial message } void loop() { if(Serial.available()>0){ //checks if something is recieved from processing char inByte=Serial.read(); //and reads it int photoCellReading = analogRead(A0); //reads my photocell delay(1); Serial.println(photoCellReading); //sends it to processing } }
Processing Code:
import processing.serial.*; Serial myPort; int data=0; int userInputCategory = 0; //variable dependent on user input int correctCategory; //variable dependent on location distance, used to compare with user input int randomLocation; //random location for each run //arrays of locations and how far they are from campus String[] locations = {"Louvre Abu Dhabi", "Warehouse 421", "Abu Dhabi Cultural Foundation", "Etihad Modern Art Gallery", "Alserkal Avenue", "Sharjah Art Foundation"}; int [] distanceFromCampus = {6, 10, 13, 18, 132, 167}; //loading images and font PImage image; PFont f; void setup() { size(960, 540); printArray(Serial.list()); String portname=Serial.list()[2]; println(portname); myPort = new Serial(this, portname, 9600); myPort.clear(); //clearing port from any previous data myPort.bufferUntil('\n'); // reading until the new line randomLocation = (int)random(0,locations.length); // generating a random location for the play //loading the suitable image image = loadImage(locations[randomLocation] + ".jpg"); imageMode(CENTER); image.resize(350,350); //font f = createFont("Montserrat-BoldItalic.ttf",20); textFont(f); textMode(CENTER); } void draw() { background(102,133,147); fill(0); // if statement to set the correct category based on location if (distanceFromCampus[randomLocation] <= 10) { correctCategory = 1; } else if (distanceFromCampus[randomLocation] >= 10 && distanceFromCampus[randomLocation] <= 20) { correctCategory = 2; } else if (distanceFromCampus[randomLocation] > 100) { correctCategory = 3; } //setting user input categories if (data >= 100 && data <= 200) { userInputCategory = 1; } else if (data >= 700 && data <= 830) { userInputCategory = 3; } else if (data > 855) { userInputCategory = 2; } //println(randomLocation); switch (randomLocation) { case 0: //display stuff textSize(40); text(locations[randomLocation],width/2-250, height - 450); image(image, width/2,height/2 + 30); //compares user input to the correct value and displays correct if the user is right if (userInputCategory == correctCategory) { background(102,133,147); text("CORRECT", width/2-100,height/2); break; } case 1: textSize(40); text(locations[randomLocation],width/2-250, height - 450); image(image, width/2,height/2 + 30); if (userInputCategory == correctCategory) { background(102,133,147); text("CORRECT", width/2-100,height/2); break; } case 2: textSize(40); text(locations[randomLocation],width/2-250, height - 450); image(image, width/2,height/2 + 30); if (userInputCategory == correctCategory) { background(102,133,147); text("CORRECT", width/2-100,height/2); break; } case 3: textSize(40); text(locations[randomLocation],width/2-250, height - 450); image(image, width/2,height/2 + 30); if (userInputCategory == correctCategory) { background(102,133,147); text("CORRECT", width/2-100,height/2); break; } case 4: textSize(40); text(locations[randomLocation],width/2-250, height - 450); image(image, width/2,height/2 + 30); if (userInputCategory == correctCategory) { background(102,133,147); text("CORRECT", width/2-100,height/2); break; } case 5: textSize(40); text(locations[randomLocation],width/2-250, height - 450); image(image, width/2,height/2 + 30); if (userInputCategory == correctCategory) { background(102,133,147); text("CORRECT", width/2-100,height/2); break; } } } void serialEvent(Serial myPort) { //recieves the value as string and reads it one line at a time String s=myPort.readStringUntil('\n'); s=trim(s); //trimming for any additional or hanging white spaces if (s!=null) { // if something is received data=(int (s)); //set the variable to the integer value of the string } //println(data); myPort.write('0'); //send something back to arduino to complete handshake }