I struggled to think of any creative ideas at first and just started working. After a while, my initial idea was to have 4 buttons and 4 bulbs, where clicking on a button triggers a temporary lighting of the bulbs in the order 1234, the second triggers them in the same order with the last bulb off (123), third lights 2 bulbs (12), and the fourth lights 1. If you click two times, the order changes to 4123, and the same logic applies to buttons, 2, 3, and 4. Then if three clicks it is 3412, and if 4 clicks it is 2341, basically shifting every number to fit in all four possible positions.
Major Struggles
This assignment was actually very challenging for me for several reasons. Firstaly, I was not able to get buttons until Sunday night which made me very stressed while working during the tight time. I was not able to find big colorful buttons like the ones which my peers have in their kits; I found much smaller ones instead which are all just black. This in itself made it very hard to try them out properly and I would sometimes cause the buttons to fall off from the circuit if I simply press a bit hard. In order to resolve the color issue, I actually used nail polish to color the small surfaces of the buttons.
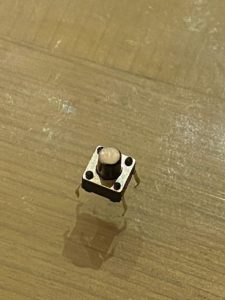
I kept working for hours and a recurring issue kept happening; anything I use in the lower half of the board simply did not work, even if I copy the literal code for it and corresponding electrical wiring from above. After hours of extreme frustration, I realized that half of my breadboard doesn’t work properly.
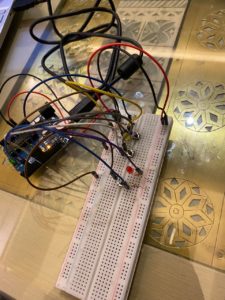
In order to resolve the issue, I actually extended my power and ground wires to the right side too, and added a button and bulb on the right side. Even though I wanted to have more than three items at the start, that was not feasible at this point.
Idea and implementation
After getting acquainted with my board and with playing with electrical tools in general, I started to dive right into my idea. I realized that lighting the bulbs one after the other might be too much work that is not very necessary so I decided to just dismiss that idea. I decided pressing certain buttons would trigger certain bulbs and that pressing several buttons together would yield another result.
I was able to achieve this goal after several trials and errors where the following applies to my final project:
- Pressing the blue button on the left turns the 3 bulbs on
- Pressing it again right after turning them on turns them all off
- Pressing the purple button in the middle turns the last 2 (yellow and white) bulbs on
- Pressing it again right after turning them on turns them off
- Pressing the dark red button on the left turns the 1st and 3rd (red and white) bulbs on
- Pressing it again right after turning them on turns them off
- Pressing/holding the first and second buttons together turns only the red (1st) one on
- Pressing/holding the second and third buttons together turns only the yellow (2nd) one on
- Pressing/holding the third and first buttons together turns only the white (3rd) one on
- Pressing/holding the 3 buttons simply turns them all off
It should be noted that I made sure that if you already had some lights on and click a certain button, it adapts the bulbs to fit the appropriate output without having to turn off all of them and resetting then turning the appropriate ones on, which is so much aesthetically pleasing.
Below, I show how clicking on two buttons lights one bulb up (red in this case).
Below is the final assignment:
I learned so much from this assignment as the experience of coding and building such circuits is quite new me, and my pace is not as fast as with Processing which will require some extra effort from my end.
Here is the code I used for the circuit:
int ledRed = 5; //pin of the red bulb int ledYellow = 9; //pin of yellow bulb int ledWhite = 11; //pin of white bulb int prevButtonState1= LOW; //previous state of blue button int prevButtonState2= LOW; //previous state of purple button int prevButtonState3= LOW; //previous state of red button int ledState1; //state of red bulb int ledState2; //state of yellow bulb int ledState3; //state of white bulb int buttonBlue = 4; //pin of blue button int buttonPurple = 8; //pin of purple button int buttonRed = 10; //pin of red button void setup() { ledState1 = LOW; //initializing blue button state to not clicked ledState2 = LOW; //initializing purple button state to not clicked ledState3 = LOW; //initializing red button state to not clicked pinMode(ledRed, OUTPUT); //declaring red led as output variable pinMode(buttonBlue, INPUT); //declaring blue button as intput variable pinMode(ledYellow, OUTPUT); //declaring yellow led as output variable pinMode(buttonPurple, INPUT); //declaring purple button as intput variable pinMode(ledWhite, OUTPUT); //declaring white led as output variable pinMode(buttonRed, INPUT); //declaring red button as intput variable Serial.begin(9600); } void loop() { int currentButtonState1 = digitalRead(buttonBlue); //setting variable to digital reading of state of blue button int currentButtonState2 = digitalRead(buttonPurple); //setting variable to digital reading of state of purple button int currentButtonState3 = digitalRead(buttonRed); //setting variable to digital reading of state of red button if ((currentButtonState1 == HIGH) && (currentButtonState2 == HIGH) && (currentButtonState3 == HIGH)) { //if all buttons are pressed, turn all bulbs off ledState1 = LOW; ledState2 = LOW; ledState3 = LOW; } else if ((currentButtonState1 == HIGH) && (currentButtonState2 == HIGH)) { //if both the blue and purple buttons are pressed/held together if (ledState1 == HIGH) { //if red bulb was lighting turn it off ledState1 = LOW; } else if (ledState1 == LOW) { //if red bulb was not on, turn it on ledState1 = HIGH; } ledState2 = LOW; //ensure the yellow bulb is off ledState3 = LOW; //ensure the white bulb is off } else if ((currentButtonState2 == HIGH) && (currentButtonState3 == HIGH)) { //if both the purple and red buttons are pressed/held together if (ledState2 == HIGH) { //if yellow bulb was on, turn off ledState2 = LOW; } else if (ledState2 == LOW) { //if yellow bulb was off, turn on ledState2 = HIGH; } ledState1 = LOW; //ensure red bulb is off ledState3 = LOW; //ensure white bulb is off } else if ((currentButtonState1 == HIGH) && (currentButtonState3 == HIGH)) { //if both the blue and red buttons are pressed/held together if (ledState3 == HIGH) { //if white bulb is on, turn off ledState3 = LOW; } else if (ledState3 == LOW) { //if white bulb is off, turn on ledState3 = HIGH; } ledState1 = LOW; //ensure red bulb is off ledState2 = LOW; //ensure yellow bulb is off } else if (currentButtonState1 == HIGH && prevButtonState1 == LOW) { //if blue button is pressed if (ledState1 == HIGH && ledState2 == HIGH && ledState3 == HIGH) { //if this was the last button previously pressed, turn off all bulbs ledState1 = LOW; ledState2 = LOW; ledState3 = LOW; } else { //otherwise, turn them all on ledState1 = HIGH; ledState2 = HIGH; ledState3 = HIGH; } } else if (currentButtonState2 == HIGH && prevButtonState2 == LOW) { //if the purple button is pressed if (ledState2 == HIGH && ledState3 == HIGH && ledState1 == LOW) { //if this was the last pressed button, turn the lit ones off ledState2 = LOW; ledState3 = LOW; } else { //otherwise, turn the yellow and white bulbs only on ledState2 = HIGH; ledState3 = HIGH; ledState1 = LOW; } } else if (currentButtonState3 == HIGH && prevButtonState3 == LOW) { //if the red button is pressed if (ledState1 == HIGH && ledState3 == HIGH && ledState2 == LOW) { //if it was teh last pressed button, turn lit ones off ledState1 = LOW; ledState3 = LOW; } else { //otherwise, turn the red and white bulbs on ledState3 = HIGH; ledState1 = HIGH; ledState2 = LOW; } } digitalWrite(ledRed, ledState1); digitalWrite(ledYellow, ledState2); digitalWrite(ledWhite, ledState3); //update the bulbs accordingly prevButtonState1 = currentButtonState1; prevButtonState2 = currentButtonState2; prevButtonState3 = currentButtonState3; //update the current states of the buttons for evaluation in the next frame }