Intro
Last week was quite a reflective week for me. I spent a lot of time thinking about how 2020 has been so far. Sure, it kind of sucks for almost all of us, but what I didn’t realize is that it’s going much faster than I ever anticipated and that there’s so much I’m grateful for this year. So, in some way, looking back feels like a blur…I remember everything and nothing at once, and I wanted to use this week’s assignment to communicate that state of mind.
Concept
The analogy I made in my brain was: looking back at the year is like watching my memories through a glitchy old TV, and so that’s exactly what I went for!
I usually create collections of 10-20 photos that represent every month for me, so I picked one picture from each month of 2020 so far to be displayed through the old tv.
Process
For starters, my first step was collecting my pictures and editing them all to be of the same dimensions and making sure these dimensions go well with the TV frame I had loaded onto my program.
Then, I started putting my images in a “slideshow’ that updates as the program is running. So, I created an array of Images and variables for slide number, Time, and slide duration and looped through my images in the draw() loop.
Afterward, I started experimenting with possible glitches. I looked at the Processing reference and examples online to come up with interesting glitches that simulate a broken TV. I placed each glitch in its own function and used a random variable to call a different glitch each run to give the sketch a more authentic feel.
Glitch 1
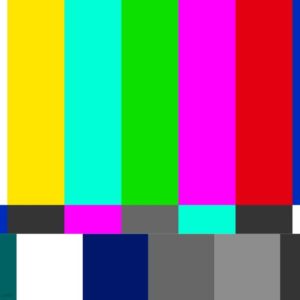
I loaded a no signal image into the sketch and wanted to overlay it onto the pictures I was loading, but I didn’t want it to be too overpowering. So, I explored the blend() function and used the MULTIPLY filter which gave me a realistic result.
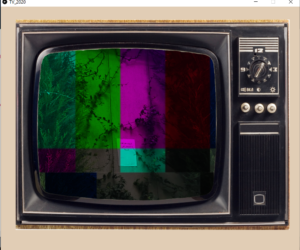
Here’s an example of what the function produces when the program runs.
void glitch1() { image(months[currentSlide], width/2-80, height/2-60); blend(noSignal, width/2-80-300, height/2-60-300, 600, 600, width/2-80-300, height/2-60-300, 600, 600, MULTIPLY); }
Glitch 2
My second glitch made use of the pixel array. I loaded the pixels for each image I used and set the color for each. For the Y value, I used a random function to create a bleeding effect so it doesn’t cover the whole TV when it alternates glitches, I also felt like it looked more interesting.
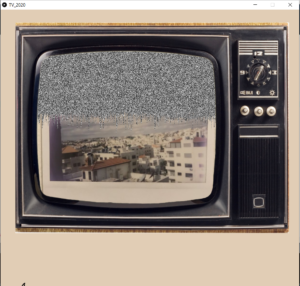
void glitch2() { months[currentSlide].loadPixels(); for (int x = 0; x < months[currentSlide].width; x++) { for (int y = 0; y < random(months[currentSlide].height-400, months[currentSlide].height); y++) { float pix = random(255); months[currentSlide].pixels[x+y*months[currentSlide].width] = color(pix); } } updatePixels(); }
Glitch 3
Here, I used the logic of getting subsections from the main image. I grabbed one fixed subsection (of the middle part always to keep the most important parts of the picture, the center, visible), and two random subsections. I placed these subsections at a random location and used the INVERT filter to create something more visually appealing. I used a modulus loop to slow down this movement. Otherwise, the program would run too fast making the glitch barely visible.
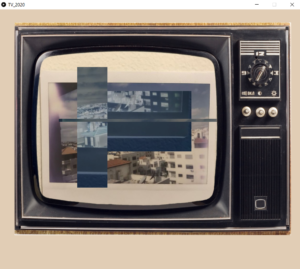
void glitch3() { pushStyle(); tint(255, 20); if (time%3== 0) { PImage subSection = months[currentSlide].get(300, 370, 500, 200); PImage subSection2 = months[currentSlide].get((int)random(0, 550), (int)random(0, 550), 100, 400); PImage subSection3 = months[currentSlide].get((int)random(0, 550), (int)random(0, 550), 900, 10); subSection.filter(INVERT); subSection2.filter(INVERT); subSection3.filter(INVERT); tint(200,255); image(subSection, random(300,722), random(140,640)); image(subSection2, random(300,722), random(270,500)); image(subSection3, random(500,722), random(270,500)); //image(subSection3, random(width/2-80-100, width/2-80), random(height/2-60-200, height/2-30)); } popStyle(); }
Glitch 4
Glitch 4 isn’t really a glitch, I just included a function that loads the image normally as I do at the beginning of the program to maintain some balance in the animation.
void glitch4() { image(months[currentSlide], width/2-80, height/2-60); }
Text
Finally, I thought I’d give the images some context. So I created a string array that contains one thing I’m grateful for each month, and the program uses the slide number to load the matching array.
Final Product
What I was prioritizing this week, is approaching the assignment systematically and in an organized manner. I often feel like I am overwhelmed with ideas and don’t know where to start, I decided to take it step by step this week and did my assignment in separate programs that I put together in the end. This made me feel productive and made the process much more enjoyable. This is one of the reasons this has been my favorite assignment so far, but I think it’s also because I enjoy working with visuals and mixing effects to achieve a specific aesthetic!
For further development, I want to familiarize myself more with the pixel array and explore the possibilities of using it. I would also be interested to see if I can generate pixels for a text if I used the geomerative library to get the points of my string.
Finally, here’s my complete code:
PImage tvFrame; PImage noSignal; PImage months[]; String gratefulFor[]; PFont f; int picsNumber; int time = 0; int slideDuration = 100; int currentSlide = 0; void setup() { size(1000, 1000); f = createFont("Nocturne-Rough.otf",60); textFont(f); tvFrame = loadImage("tv_frame.png"); tvFrame.resize(1000, 760); noSignal = loadImage("noSignal.jpg"); noSignal.resize(500, 500); picsNumber = 9; months = new PImage[picsNumber]; months[0] = loadImage("jan.JPG"); months[1] = loadImage("feb.JPG"); months[2] = loadImage("march.jpg"); months[3] = loadImage("april.jpg"); months[4] = loadImage("may.JPG"); months[5] = loadImage("june.jpg"); months[6] = loadImage("july.JPG"); months[7] = loadImage("aug.JPG"); months[8] = loadImage("sept.jpg"); for (int i = 0; i < months.length; i++) { months[i].resize(600, 600); } gratefulFor = new String[picsNumber]; gratefulFor[0] = "January: travel"; gratefulFor[1] = "February: live music"; gratefulFor[2] = "March: safety"; gratefulFor[3] = "April: windows"; gratefulFor[4] = "May: family"; gratefulFor[5] = "June: archives/memories"; gratefulFor[6] = "July: nature"; gratefulFor[7] = "August: loss"; gratefulFor[8] = "September: friendship"; } void draw() { background(225, 205, 181); imageMode(CENTER); image(months[currentSlide], width/2-80, height/2-60); fill(0); textSize(50); text(gratefulFor[currentSlide], width/2 - 450, height/2 + 450); time+=1; if (time>slideDuration) { currentSlide +=1; if (currentSlide > months.length-1) { currentSlide = 0; } time = 0; } int glitchNo = (int)(random(1, 5)); if (glitchNo == 1) { glitch1(); } else if (glitchNo ==2) { glitch2(); } else if (glitchNo == 3) { glitch3(); } else if (glitchNo == 4) { glitch4(); } image(tvFrame, width/2, height/2-100); //println(glitchNo); } void glitch1() { image(months[currentSlide], width/2-80, height/2-60); blend(noSignal, width/2-80-300, height/2-60-300, 600, 600, width/2-80-300, height/2-60-300, 600, 600, MULTIPLY); } void glitch2() { months[currentSlide].loadPixels(); for (int x = 0; x < months[currentSlide].width; x++) { for (int y = 0; y < random(months[currentSlide].height-400, months[currentSlide].height); y++) { float pix = random(255); months[currentSlide].pixels[x+y*months[currentSlide].width] = color(pix); } } updatePixels(); } void glitch3() { pushStyle(); tint(255, 20); if (time%3== 0) { PImage subSection = months[currentSlide].get(300, 370, 500, 200); PImage subSection2 = months[currentSlide].get((int)random(0, 550), (int)random(0, 550), 100, 400); PImage subSection3 = months[currentSlide].get((int)random(0, 550), (int)random(0, 550), 900, 10); subSection.filter(INVERT); subSection2.filter(INVERT); subSection3.filter(INVERT); tint(200,255); image(subSection, random(300,722), random(140,640)); image(subSection2, random(300,722), random(270,500)); image(subSection3, random(500,722), random(270,500)); //image(subSection3, random(width/2-80-100, width/2-80), random(height/2-60-200, height/2-30)); } popStyle(); } void glitch4() { image(months[currentSlide], width/2-80, height/2-60); }
I love this!!! Such a cool idea!
Thank you so much Ons!!<3
I loveee this, so wholesome! And the TV says “ВКЛ” (“on”) in russian haha
Thank youuuu Amina! and thank you for pointing the Russian out, I didn’t notice it at first 🙂
Really great work Sarah from every perspective. It’s great how you had a concept, tied it into your already existing life (art) practice, searched out new programming techniques you needed, incorporated it all together, focusing on the aesthetics of want you wanted to express, and made something really well put together. Bravo!
Thank you so much Aaron!! Working on this was very enjoyable.