Concept:
Upon looking at a bunch of open source data sets, I found a data set for Exoplanets that have been documented outside our solar system by NASA: https://www.kaggle.com/datasets/adityamishraml/nasaexoplanets
I thought this would be pretty cool to implement as a form of data visualization as I don’t think I’ve seen any examples of data visualization for astronomy or space hence, I decided to implement this into my assignment.
Process and Planning:
For visualizing the exoplanets, I didn’t want to simply draw an ellipse as I thought it wouldn’t look that great and it would be too simple. Hence, I found some nice planet art online and used some of them to visualize some of the exoplanets:
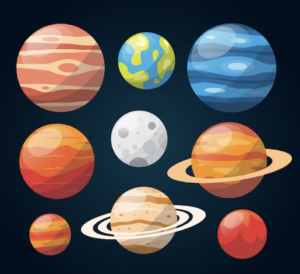
I used a few of these planets and put them into some img variables and then put them all into an array of planet designs so that I could access any design I wanted from this array anytime I wanted. I also loaded the entire exoplanets.csv dataset into an array called exoplanet_data which I would plan to use later in my code.
function preload() {
exoplanet_data = loadStrings("exoplanets.csv");
planet1_img = loadImage("Planet1.png");
append(planet_designs, planet1_img);
planet2_img = loadImage("Planet2.png");
append(planet_designs, planet2_img);
planet3_img = loadImage("Planet3.png");
append(planet_designs, planet3_img);
planet4_img = loadImage("Planet4.png");
append(planet_designs, planet4_img);
planet5_img = loadImage("Planet5.png");
append(planet_designs, planet5_img);
}
I knew that I would have to create a class in which each exoplanet would be its own object, hence I made one here:
class ExoPlanet {
constructor() {
this.x; //X coordinate for the planet
this.y; //Y coordinate for the planet
this.planet_radius; //Radius of the planet image
this.design_num; //Type of planet design from planet_designs
this.data; //String data of the planet
this.data_split; //Splits the data into an array of its data
this.init();
this.printData();
}
//This function initializes all the variables for each exoplanet.
init() {
this.design_num = int(random(5));
this.planet_radius = planet_designs[this.design_num].width/10;
this.x = mouseX;
this.y = mouseY;
this.data = exoplanet_data[data_count];
this.data_split = split(this.data, ',');
if(data_count != exoplanet_data.length) {
data_count++;
}
else {
print("All planets printed!");
}
}
//This function takes a random planet image and draws it at a random (x,y) coordinate on the canvas
draw() {
image(planet_designs[this.design_num], this.x, this.y, planet_designs[this.design_num].width/5, planet_designs[this.design_num].height/5);
}
displayData() {
fill(255, 255, 180);
rect(mouseX, mouseY, 500, 120);
textAlign(LEFT);
fill("black");
text("Planet Name:", mouseX+10, mouseY+30);
text("Distance from Earth (ly):", mouseX+10, mouseY+55);
text("Stellar Magnitude (brightness):", mouseX+10, mouseY+80);
text("Planet Type:", mouseX+10, mouseY+105);
fill("red");
text(this.data_split[0], mouseX+140, mouseY+30);
text(this.data_split[1], mouseX+235, mouseY+55);
text(this.data_split[2], mouseX+290, mouseY+80);
text(this.data_split[3], mouseX+125, mouseY+105);
}
//This function is for debugging and code checking to ensure everything runs smoothly.
printData() {
print("Planet Image:",this.design_num);
print("Planet Radius:",this.planet_radius);
print("Planet Data:",this.data);
print("Planet Name:",this.data_split[0]);
print("Distance (from Earth):",this.data_split[1]);
print("Stellar Magnitude:",this.data_split[2]);
print("Planet Type:",this.data_split[4]);
}
}
In this class, each planet has:
- An (X, Y) coordinate of the planet on the canvas (this would be where the user’s mouse is when they click)
- The radius of the planet (so when the user moves close to the planet, its data pops up)
- Design number: This indicates any one of the planet images shown perviously.
- Data: This entails the particular planet data from the csv file for that planet.
- Data_Split: This variable would be an array of each component of the data of the planet, hence, it would be easier to print out its data.
I had an initialize function, init(), to initialize it with some variables. I had another function which would draw the planet onto the canvas using the image(). Finally, I had a displayData() function which would print out a rectangle containing some of the vital information of the exoplanet’s data.
How does the data pop up?
//Here we continuously draw the planets on the canvas
for(let j=0; j<exoplanets.length; j++) {
exoplanets[j].draw();
planet_dist = dist(exoplanets[j].x, exoplanets[j].y, mouseX, mouseY);
//If the mouse coordinates hit's the planet, it will print the data of that planet.
if(planet_dist < exoplanets[j].planet_radius) {
exoplanets[j].displayData();
}
}
Essentially, in the draw() function, it will continuously print the exoplanet using the draw function from the class. It calculates the planet_dist between the planets coordinates and the user’s mouse. If the user’s mouse touches the planet, then it will call the displayData() function.
I added it such that the user can add a planet onto the canvas by simply clicking their mouse as well.
Final Piece:
Overall Thoughts:
Overall, I’m pretty happy with the interactivity and design with my assignment. Perhaps, for improvements I could have included more planet shapes, and maybe even added some cool sound effects to enhance the experience a little more. More improvements, I could have also made sure that if the planet size was bigger, the planet drawn could also be bigger and vice versa. This way the data visualization is even stronger.
Midterm Project Ideas:
For my midterm, I wanted to develop a game since I really love games and would love to get into game development. Some of the ideas that initially sparked in my head was perhaps something like “Battleship” or maybe something like a parody of “Subway Surfers” where the user can continuously run and get the highest score or something like that. For now these are the ideas I thought of initially but I’d love to come up with a cool unique game of my own.