Intro
Starting something new is always scary. Ever since class on Wednesday, I had this assignment on my mind and I kept questioning how fast I could be able to get accustomed to working with the Arduino Uno. After going through our class recording and playing around with the elements a bit, I felt nostalgic for the days I did Lego robotics in high school and realized that it’s a lot of fun exploring the possibilities that this kit has. Also, I felt like getting feedback from something you’re doing with your hands when you’re trying to debug your code is so helpful! It helped me understand better how what I write is translating into reality.
Idea
So, my first instinct was to create a puzzle based on color theory. Maybe that the user needs to press the yellow and blue buttons to turn on an LED? So I went down that path and decided to make a puzzle that’s inspired by the colors of the rainbow.
Game Play
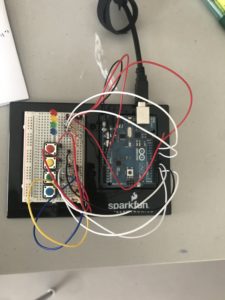
A player has 4 buttons and 4 LEDs in front of them, and the goal is to get all four LEDs flashing simultaneously. Before starting they get a few instructions or pointers:
- You need six steps to achieve the task
- These steps have a specific order/sequence
- You can press a maximum of two buttons at the same time
- Here’s a riddle to give you a hint:
“You see me in the air
But I am not a kite
I am what’s created
When water refracts light”
(I found this on a children’s riddle website)
Solution
The solution is inspired by the colors of the rainbow which I considered to be (Red, Orange, Yellow, Green, Blue, Violet) ((Sorry, Indigo)). So here are the steps to the solution:
- Press Red Button
- Press Red + Yellow Buttons
- Press Yellow Button
- Press Green Button
- Press Blue Button
- Press Blue + Red Buttons
It has to be in that specific sequence, or else the LEDs won’t flash!
Video Examples
(Just realized I should’ve filmed horizontal videos!)
My solution is highly dependent on the sequence, so if the Red button is pressed in step one, the Red LED turns on to signify the completion of the step. Otherwise, it wouldn’t trigger anything. Here’s an example of me pressing some buttons at the wrong time
Finally, I thought it would be fun if I got someone to test it so my suitemate volunteered! She almost got it from the first time! 🙂
Logic
To transition between steps, I used Switch() and created a variable number called step number to move between the steps of my solution sequence. So, when step number = 0, the puzzle only responds to the play pressing the red button.
Improvement
One point of improvement could be adding another LED that flashes when a wrong button is pressed, to add more feedback for the user. I pointed out where that would be included in my code in the comments.
Also, if I had an extra button, I would make a reset button since the user has to get through the puzzle for it to restart or I have to re-upload the program.
Code
Here’s my commented code!
//introduce LED pin variables int blueLedPin = 10; int greenLedPin = 11; int yellowLedPin = 12; int redLedPin = 13; //introduce Button pin variables int redButtonPin = 7; int yellowButtonPin = 6; int greenButtonPin = 5; int blueButtonPin = 4; //introduce variable to keep track of steps int stepNo = 0; void setup() { //set pin modes pinMode(redLedPin, OUTPUT); pinMode(yellowLedPin, OUTPUT); pinMode(greenLedPin, OUTPUT); pinMode(blueLedPin,OUTPUT); pinMode(redButtonPin, INPUT); pinMode(yellowButtonPin, INPUT); pinMode(greenButtonPin, INPUT); pinMode(blueButtonPin, INPUT); Serial.begin(9600); } void loop() { //creating variables for all button states, to read if button is pressed int redButtonState = digitalRead(redButtonPin); int yellowButtonState = digitalRead(yellowButtonPin); int greenButtonState = digitalRead(greenButtonPin); int blueButtonState = digitalRead(blueButtonPin); //using a switch function to move between steps, this makes the sequence aspect of the puzzle possible. switch (stepNo) { // 1st color is red, move must be red button case 0: // checks if red button is pressed, and turns it on then off accordingly if (redButtonState == HIGH) { digitalWrite(redLedPin, HIGH); delay(700); digitalWrite(redLedPin, LOW); //if the step is performed correctly, it moves on to the next step of the puzzle stepNo += 1; //here, there's a possibility to add an else if statement, where if any of the other buttons are pressed an //additional "error" LED light would flash, indicating a mistake } break; case 1: //2nd color is orange, move must be red + yellow buttons if (yellowButtonState == HIGH && redButtonState == HIGH) { digitalWrite(redLedPin, HIGH); digitalWrite(yellowLedPin, HIGH); delay(700); digitalWrite(redLedPin, LOW); digitalWrite(yellowLedPin, LOW); stepNo += 1; } break; case 2: //3rd color is yellow, move must be yellow button if (yellowButtonState == HIGH) { digitalWrite(yellowLedPin, HIGH); delay(700); digitalWrite(yellowLedPin, LOW); stepNo += 1; } break; case 3: //4th color is green, move must be green button if (greenButtonState == HIGH) { digitalWrite(greenLedPin, HIGH); delay(700); digitalWrite(greenLedPin, LOW); stepNo += 1; } break; case 4: //5th color is blue, move must be blue button if (blueButtonState == HIGH) { digitalWrite(blueLedPin, HIGH); delay(700); digitalWrite(blueLedPin, LOW); stepNo += 1; } break; case 5: //6th color is violet, move must be blue + red buttons if (blueButtonState == HIGH && redButtonState == HIGH) { //introducing a variable for number of times that all the lights will flash, will use in a while loop int flashes = 0; //10 flashes when the user finishes the pattern while(flashes < 10){ //flashing technique from class, all LEDs on, delay, LEDs off, delay digitalWrite(blueLedPin, HIGH); digitalWrite(redLedPin, HIGH); digitalWrite(yellowLedPin, HIGH); digitalWrite(greenLedPin, HIGH); delay(400); digitalWrite(blueLedPin, LOW); digitalWrite(redLedPin, LOW); digitalWrite(yellowLedPin, LOW); digitalWrite(greenLedPin, LOW); delay(400); //adding flashes to eventually exit the while loop flashes+=1;} //restarts the puzzle stepNo = 0; } break; } }