Regarding analog sensors, I was curious how I can mimic one of the games where you have to tap countless times in order to win. At the same time, I thought of the idea that portrays a battery charging by tapping. So, the circuit I have made is a similar but simpler version where I utilized the pressure sensor and five LEDs to create the following experience for the users.
In the beginning, the problem I had was controlling the light of the LEDs. I was used to writing digitalWrite as an indicator for turning the LEDs on and off, but it did not allow me to control the faintness of the lights. After some research into Arduino, I figured out that I can manipulate the brightness of the LEDs by using analogWrite, where it would take the pin and the level of brightness (from 0 to 255) as arguments. By putting it together with the mapped value from the sensor, I was able to display a general fading of the LEDs.
However, the problem did not stop here. Through user testing, I realized that people can lit up the LEDs by just pressing onto the pressure sensor. So, I made a change to use the computedPressureValue, the difference between current value from the pressure sensor to the previous value, to increment the brightness. When the pressure difference is negative, meaning that the previous value is larger than the current value, it meant that the pressure is decreasing and the finger is lifted off from the sensor. Thus, only tapping on the sensor will slowly brighten the LEDs.
Below are the snapshot of the circuit, a run-through of the experience, and the code for the circuit.
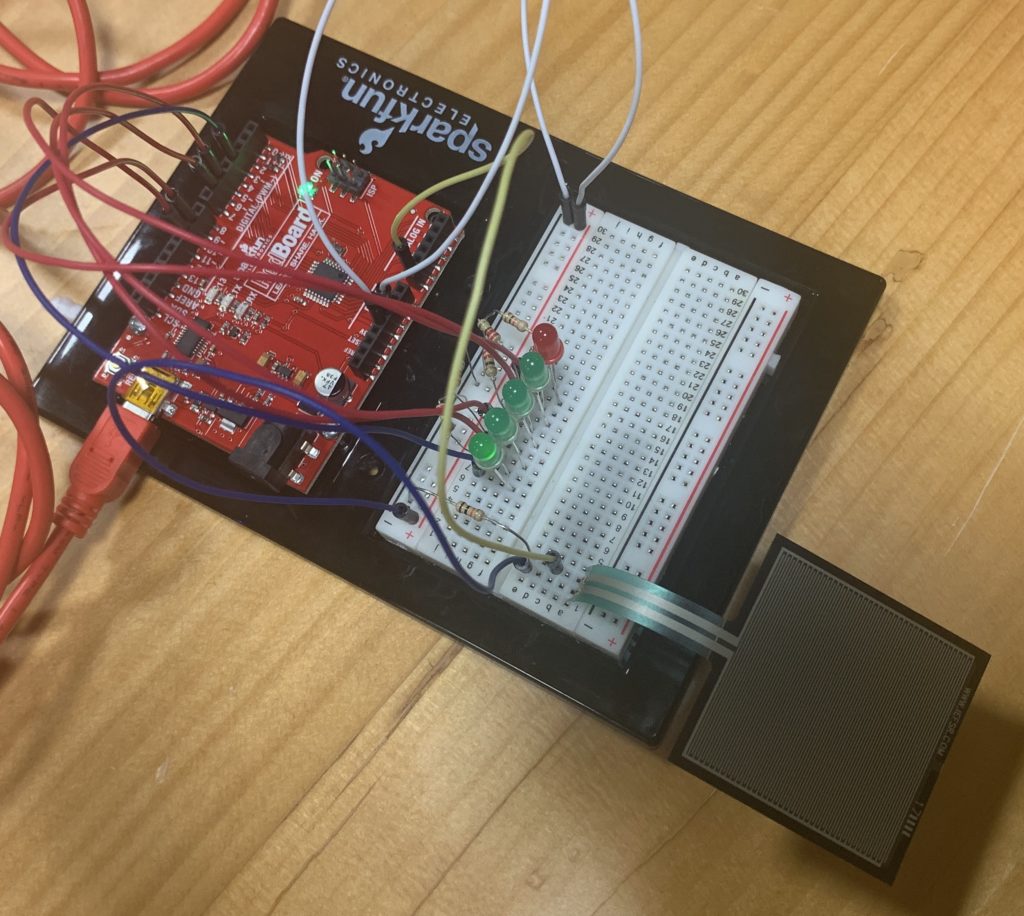
// Variables for LED Pin Outputs const int ledPinOne = 3; const int ledPinTwo = 5; const int ledPinThree = 6; const int ledPinFour = 9; const int ledPinFive = 10; // Variables for LED States bool ledStateOne = true; bool ledStateTwo = false; bool ledStateThree = false; bool ledStateFour = false; bool ledStateFive = false; bool ledStateComplete = false; // Number of LED that the has lit up int ledCount = 0; // The number of blinking of LEDs after all five LEDs has lit up int completedTask = 0; // Previous value of the readings for the pressure sensor int prevPressureValue = 0; // Raw value for brightness int brightness = 0; void setup() { pinMode(ledPinOne, OUTPUT); pinMode(ledPinTwo, OUTPUT); pinMode(ledPinThree, OUTPUT); pinMode(ledPinFour, OUTPUT); pinMode(ledPinFive, OUTPUT); Serial.begin(9600); } void loop() { // ---- COMPUTATION OF VARIABLE ---- // // The brightness is computed by using a modulus of 200 so that it has the max-limits of 200. int computedBrightness = brightness % 200; int pressureValue = analogRead(A0); // The pressure sensor has a min. and max. values of 0 and 1010, which I have mapped for conversion from 0 to 100. int currentPressureValue = map(pressureValue, 0, 1010, 0, 100); // In order to increase the brightness for each tabs, I have used to difference of current and previous pressure values. int triggerPressureValue = currentPressureValue - prevPressureValue; if (triggerPressureValue < -10) { brightness += 2; } // ---- CONDITIONAL CHECKS FOR LED STATES & BRIGHTNESS ---- // if (ledStateFive) { analogWrite(ledPinFive, computedBrightness); if (computedBrightness > 190) { ledStateFive = false; ledCount += 1; } } if (ledStateFour) { analogWrite(ledPinFour, computedBrightness); if (computedBrightness > 190) { ledStateFour = false; ledStateFive = true; ledCount += 1; brightness = 0; } } if (ledStateThree) { analogWrite(ledPinThree, computedBrightness); if (computedBrightness > 190) { ledStateThree = false; ledStateFour = true; ledCount += 1; brightness = 0; } } if (ledStateTwo) { analogWrite(ledPinTwo, computedBrightness); if (computedBrightness > 190) { ledStateTwo = false; ledStateThree = true; ledCount += 1; brightness = 0; } } if (ledStateOne) { analogWrite(ledPinOne, computedBrightness); if (computedBrightness > 190) { ledStateOne = false; ledStateTwo = true; ledCount += 1; brightness = 0; } } // ---- THE PROCESS AFTER ALL FIVE LEDS ARE LIT UP ---- // if (ledCount == 5) { brightness = 0; while (completedTask < 10) { for (int i=0; i<6; i++) { analogWrite(ledPinOne, brightness); analogWrite(ledPinTwo, brightness); analogWrite(ledPinThree, brightness); analogWrite(ledPinFour, brightness); analogWrite(ledPinFive, brightness); brightness += 18; delay(20); } for (int i=0; i<6; i++) { analogWrite(ledPinOne, brightness); analogWrite(ledPinTwo, brightness); analogWrite(ledPinThree, brightness); analogWrite(ledPinFour, brightness); analogWrite(ledPinFive, brightness); brightness -= 18; delay(20); } completedTask += 1; } // Reset variable values to repeat the experience completedTask = 0; ledCount = 0; brightness = 0; ledStateOne = true; analogWrite(ledPinOne, 0); analogWrite(ledPinTwo, 0); analogWrite(ledPinThree, 0); analogWrite(ledPinFour, 0); analogWrite(ledPinFive, 0); } // ---- SETTING PREVIOUS PRESSURE VALUE WITH THE CURRENT VALUE BEFORE NEW LOOP ---- // prevPressureValue = currentPressureValue; }