Exercise 1
Scheme: 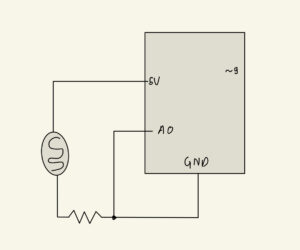
Code:
let xVal = 0; //initializing the X value for the position function setup() { createCanvas(640, 480); } function draw() { background(230); if (!serialActive) { text("Press Space Bar to select Serial Port", 20, 30); } else { // draw an ellipse ellipse(xVal, height/2, 100, 50); //giving the ellipse the value of the x position initialized above } } function keyPressed() { if (key == " ") { // important to have in order to start the serial connection!! setUpSerial(); } } function readSerial(data) { if (data != null) { xVal = map(data, 0, 500, 0, width); //mapping the values received from the photosensor to control the X position of the ellipse console.log(data); } }
const int pSensor = A0; void setup() { pinMode(pSensor, INPUT); Serial.begin(9600); } void loop() { int val = analogRead(pSensor); //initializing the value received from the sensor Serial.println(val); //sending the value of the sensor to p5js }
Video:
Exercise 2
Scheme:
Code:
//p5js code let change = 0; //initializing the variable for the distance between two consecutive center points of the rectangle let previousX; //initializing the variable for the X dimension of the previous position of the rectangle let previousY; //initializing the variable for the Y dimension of the previous position of the rectangle function setup() { createCanvas(640, 480); textSize(18); textAlign(CENTER); previousX = 0; previousY = 0; } function draw() { background('red'); if (!serialActive) { fill('white'); text("Press Space Bar to select Serial Port", width/2, height/2); } else { fill('white'); rectMode(CENTER); rect(mouseX, mouseY, 200,200); //controlling the center of the rectangle with the mouse fill('black'); text("Move as fast as you can to control the brightness of the LED", width/2,20); getChange(); //calling the function that will track the distance between the two consecutive positions of the rectangle } } //calculating the distance of two consecutive rectangle positions function getChange(){ change = floor(sqrt(pow((mouseX - previousX),2) + pow((mouseY - previousY),2))); previousX = mouseX; previousY = mouseY; } function keyPressed() { if (key == " ") { setUpSerial(); } } function readSerial(data) { if (data != null) { console.log(change); writeSerial(change); //sending the value of the distance two control the LED brightness } }
const int led = 9; void setup() { // put your setup code here, to run once: pinMode(led, OUTPUT); Serial.begin(9600); while (Serial.available() <= 0) { Serial.println("0"); delay(300); } } void loop() { while (Serial.available()) { int brightness = Serial.parseInt(); // read the incoming data as an integer analogWrite(led, brightness); // set the LED brightness based on incoming data Serial.println(); } }
Video:
Exercise 3
Scheme: 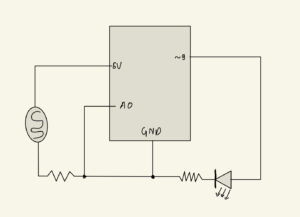
Code:
//p5js code let velocity; let gravity; let position; let acceleration; let wind; let drag = 0.99; let mass = 50; let ard_wind = 1; //initializing the wind speed let bit; function setup() { createCanvas(640, 360); position = createVector(width / 2, 0); velocity = createVector(0, 0); acceleration = createVector(0, 0); gravity = createVector(0, 0.5 * mass); wind = createVector(0, 0); bit = 0; //initializing the value for the LED } function draw() { background(255); if (!serialActive) { text("Press Space Bar to select Serial Port", 20, 30); } else { applyForce(wind); applyForce(gravity); velocity.add(acceleration); velocity.mult(drag); position.add(velocity); acceleration.mult(0); ellipse(position.x, position.y, mass, mass); if (position.y > height - mass / 2) { velocity.y *= -0.9; // A little dampening when hitting the bottom position.y = height - mass / 2; bit = 1; //lighting up the LED upon collision with "the ground" } else { bit = 0; //turning off the LED when the ball is not touching "ground" } } } function applyForce(force) { // Newton's 2nd law: F = M * A // or A = F / M let f = p5.Vector.div(force, mass); acceleration.add(f); } function keyPressed() { if (keyCode == LEFT_ARROW) { wind.x = ard_wind * -1; //when left arrow is pressed, the velocity of the wind attains a negative value and the ball moves to the left } if (keyCode == RIGHT_ARROW) { wind.x = ard_wind; //when the right arrow is pressed, the velocity of the wind attains a positive value and the ball moves to the right } if (keyCode == DOWN_ARROW) { mass = random(15, 80); position.y = -mass; velocity.mult(0); } if (key == " ") { // important to have in order to start the serial connection!! setUpSerial(); } } function readSerial(data) { if (data != null) { let sen = bit + "\n"; writeSerial(sen); //sending the values to light up or turn off the LED ard_wind = map(data, 0, 300, 0, 10); // mapping the data received from arduino to a sensible range for the wind velocity console.log(ard_wind); } }
const int led = 12; const int photoSensor = A0; void setup() { // put your setup code here, to run once: Serial.begin(9600); pinMode(led, OUTPUT); pinMode(photoSensor, INPUT); while (Serial.available() <= 0) { Serial.println(0); // delay(300); } } void loop() { while (Serial.available() > 0) { int bit = Serial.parseInt(); // read the incoming data as an integer digitalWrite(led, bit); // set the LED brightness based on incoming data //send the values of the photosensor to p5js int val = analogRead(photoSensor); Serial.println(val); } }
Video: