If you keep watching the numbers until they fill up the whole canvas, you will see a surprise!
The concept I was going for in this assignment was Matrix themed. Originally, I wanted to have a different work of art for “out of the Matrix” and “stay in the Matrix”, but I ended up just doing something simple instead.
Process + Highlight
The whole process of making this was similar to the self portrait; there was a lot of trial and error. Essentially, I just experimented until I got something I liked.
I started off with a black background and added green ellipses that were slightly transparent. I later changed it to rectangles. I tested different transparencies and sizes before I settled for the final look. random() is my favorite method to use because I don’t have to make a choice and it’s interesting to see how the “art” changes each time you run it (also you will see how often I use random() in this assignment).
function matrixNumbers() { fill(0, 143, 17); textFont("Courier New"); textSize(20); if(random(2) < 1) { text("0", textPosX, textPosY + spacing); } else { text("1", textPosX + spacing, textPosY); } textPosY = textPosY + spacing; if (textPosY > height) { textPosY = 0; textPosX = textPosX + spacing; } }
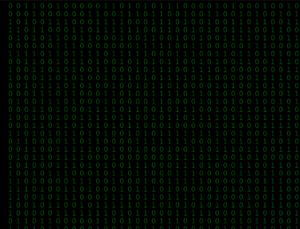
The one thing I was certain I was going to do was the numbers appearing down the screen. At first, I had the numbers in an organized fashion by having textPosX + spacing
and textPosY + spacing
in both the if and else statements. Then I changed it to the code above, which staggered and stacked the numbers, which I felt was more interesting than a grid-like fashion. I also realized that globalizing the variables will create the dynamic aspect of the numbers showing up one by one. At first I just put the variables in the function, but this just stacked 0 and 1 in the corner (I think this happens because when you keep it in the function it changes once, whereas when you globalize it, the variable changes every time the function is called in draw?)
This code was actually taken from a previous p5 assignment I did, but I modified it to fit the Matrix number pattern:
A 10Print assignment I did for another class
This isn’t really related to loops, but I really like how the pills appeared after the numbers finish appearing on the screen. I experimented with setAlpha() to make the pills and text appear gradually. I got this code from the reference, but I modified it because I didn’t need it to go constantly go from opaque to transparent. Originally, I followed the reference and did sin(millis() / 1000)
, but it didn’t work the way I wanted it to, so I looked at the reference for just millis() and realized that I didn’t need the sin().
I wanted the red pill and blue pill to contrast each other in terms of the shapes used. So for the red pill, I wanted to use arcs, but I didn’t really like how the empty space looked, so I used circles and adjusted the transparency. For the blue pill, I wanted it to be like pixel art. Again, I used random so the colors would be different each time. I originally had the squares larger, but the smaller it was, the more intense the pixel effect was so I kept it small.
Future Improvements and Ideas
Currently, I’m kind of cheating with how the red pill and blue pill works. This is the code:
if (mouseIsPressed && mouseX < width / 2) { noLoop(matrixNumbers()); redPill(); } if (mouseIsPressed && mouseX > width / 2) { noLoop(matrixNumbers()); bluePill(); }
So no matter where on the canvas, as long as it’s less than half of the width, it will take you to the red pill. Similarly for the blue pill, as long as it’s more than half of the canvas width it will change. Therefore, even before the pills appear you can click to see either the red pill or blue pill “art”. I was thinking of using dist(), but I was a bit confused as to how with a rectangle. Additionally, since my code doesn’t include a way to go back to the numbers screen, once you click on one side you would have to restart the program in order to see the other pill. I’d like to say this was an intentional design because once you pick one, you can’t go back. However, since the mouse press range isn’t only within the two pills, if you accidentally click somewhere it will take you to either pill drawings.
I want to add more dimension to the red pill and blue pill drawings, such as lines or curves drawing over it, similar to what Casey Reas does. Actually, the more I look at the arcs version of the red pill art, the more I like how random it is. So another future idea might be combining the current, organized version with the arcs. For example, make the circles change into arcs using millis() and loop that, similar to what the reference for setAlpha() does.