Overview:
Finally, it’s here.
The purpose of this game is for the student, skeleton in the picture, to collect all the questions and then collect the final paper to get the full grade for their exam.
IDEA(details):
Background:
Originally, I intended to have clouds as the background with the stars, but even when I used images instead of pixels, the game’s frameRate dropped and the game was barely functioning so I settled for a clear starry night look.
I choose this because:
firstly, I have really sensitive eyes, and I find it easier on my eye to look at a dark screen.
Secondly, I am a night person, night always looks better ;).
For the stars, I used overlapping circles to give a faded look, which resembles actual stars more than normal circles would.
Code:
class Bg { float[] x = new float[50]; float[] y = new float[50]; Bg() { for (int i=0; i<50; i++) { x[i] = random(0, widthh); y[i] = random(0, heightt); } } void drawBG() { if (frameCount%50==0) { for (int i=0; i<50; i++) { x[i] = random(0, width); y[i] = random(0, height); } } background(50, 50, 80); for (int i=0; i<50; i++) { for (int j=0; j<10; j++) { noStroke(); fill(255, 25); ellipse(x[i], y[i], 1+j, 1+j); } } } }
Creature:
Instead of making repetitive classes, I used class inheritance, where I made the Superclass, Creatures, for all the live items in the game.
Code:
public class Creature { // Attributes PVector pos; PVector speed; boolean alive; float ground; PVector size; PVector imgSize; int numFrames; PImage imgs; PImage[] img; int frame; boolean dir; //left = 0 right = 1 boolean secondJump = false; public Creature(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames) { pos= new PVector(x, y); speed= new PVector(0, 0); alive = true; ground = height-g; numFrames = num_frames; imgSize = new PVector(_img.width/num_frames, _img.height); size = new PVector (r, imgSize.y*(r/imgSize.x)); imgs = _img; img = new PImage[numFrames]; for (int i=0; i<numFrames; i++) { img[i] = imgs.get( int((imgSize.x)*i), 0, int(imgSize.x), int(imgSize.y)); } // for (int y=0; y < 4; y++) { // for (int x=0; x< 12; x++) { // sprites[y][x] = spritesheet.get(x*w, y*h, w, h); // } // } frame = 0; dir = true; } void gravity() { if (pos.y<ground-size.y+10 ||pos.y<ground-size.y ) { speed.y+=0.2; } else { speed.y=0; pos.y = constrain(pos.y, ground-size.y, ground-size.y+10); secondJump=false; } for (int p=game.plats.length-1; p>=0; p--) { if ((pos.y+size.y <=game.plats[p].pos.y+15)//height && pos.x+size.x/3>=game.plats[p].pos.x//right && pos.x-size.x/3 <= game.plats[p].pos.x+game.plats[p].pWidth) {//left ground = game.plats[p].pos.y; break; } else { ground = heightt-game.ground; } } } void update() { gravity(); pos.x += speed.x; pos.y += speed.y; } void display() { update(); // println(this.getClass().getName()); if (dir && alive) { scale(1, 1); image(img[frame], pos.x, pos.y, size.x, size.y); } else if (!dir && alive) { if (this.getClass().getName()=="Midterm$Player") { pushMatrix(); scale(-1, 1); image(img[frame], -pos.x, pos.y, size.x, size.y); popMatrix(); } else if (this.getClass().getName()=="Midterm$Monster") { image(img[frame], pos.x, pos.y, size.x, size.y); } } } }
Player:
For the player, I choose the skeleton, because obviously, we are all dead during midterm and final seasons. That also gave me the idea of the name “dead student“.
Sprite:
With the player, I struggled a little with the jumps. I wanted to only allow double jumps, which I thought was the case until my roommate tried it and I realized it didn’t. I tried to fix it, but that only made it worse, so I left it as it was when my roommate tried it. (I mean what works, works right?)
Code:
class Player extends Creature { boolean left; boolean up; boolean right; SoundFile jump; int count; Player(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames, PApplet sketch) { super(x, y, r, g, _img, w, h, num_frames); left = false; up = false; right = false; jump = new SoundFile(sketch, "jump.wav"); count = frameCount; } void update() { gravity(); //==================== if (left==true) { speed.x=-3; dir=false; } else if (right==true) { speed.x=3; dir=true; } else { speed.x=0; } //==================== if (up==true && pos.y>=ground-size.y &&secondJump==false) { jump.play(); speed.y = -6; } else if (up==true && speed.y<0 && secondJump==true && pos.y<ground-size.y) { jump.play(); speed.y = -8; secondJump=false; } pos.x +=speed.x; pos.y +=speed.y; if (frameCount%5 == 0 && speed.x !=0 && speed.y ==0) { frame= (frame+1)%numFrames; } else if (speed.x==0) { frame=8; } //==================== if (pos.x<=0) { pos.x = 0; } //==================== if (pos.x+size.x>= widthh) { pos.x = widthh- size.x; } else if (pos.x<size.x) { pos.x = size.x; } //==================== for (int i =0; i<game.myMonsters.length; i++) { float numME = pow((pow(size.x, 2)+(pow(size.y, 2))), 0.5); float numthem = pow((pow(game.myMonsters[i].size.x, 2)+(pow(game.myMonsters[i].size.y, 2))), 0.5); if (game.myMonsters[i].distance(this)<=numME/2.5+numthem/2.5 && game.myMonsters[i].alive) { if (speed.y>0) { game.myMonsters[i].alive=false; game.myMonsters[i].sound2.play(); } else { game.myMonsters[i].sound1.play(); alive = false; } } } for (int i =0; i<game.myQuestions.length; i++) { if (game.myQuestions[i].distance(this)<=size.x/2+game.myQuestions[i].size.x/2 && game.myQuestions[i].alive) { game.score++; game.myQuestions[i].sound.play(); game.myQuestions[i].alive = false; } } if (game.myfinal.distance(this)<=size.x/2+game.myfinal.size.x/2 && game.myfinal.alive) { game.score+=5; game.myfinal.sound.play(); game.myfinal.alive = false; game.won= true; } } }
Monsters:
Finding Sprites for the monsters was not easy. I was trying to find monsters that represent the distractions we have while studying. So instead of finding different monsters, I made my own using emojis and clip art images.
I used the facial features from the emojis and the body of what I think represents distractions. I made them alternate between an Innocent look and an evil look because distractions usually don’t look so bad.
Here are the sprites:
Monster 1, Phone:
Monster 2, Thoughts:
Monster 3, Anxiety:
Code:
class Monster extends Creature { SoundFile sound1, sound2; float xleft, xright; Monster(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames, float x_left, float x_right, PApplet sketch) { super(x, y, r, g, _img, w, h, num_frames); speed.x=random(0.3,0.9); speed.y=0; xleft = x_left; xright = x_right; sound1 = new SoundFile(sketch, "eat.wav"); sound2 = new SoundFile(sketch, "kill.wav"); } void update() { gravity(); if (pos.x<=xleft) { dir = true; frame= (frame+1)%numFrames; speed.x*=-1; } else if (pos.x>=xright) { dir = false; frame= (frame+1)%numFrames; speed.x*=-1; } pos.x +=speed.x; pos.y +=speed.y; } float distance(Player target) { return dist(this.pos.x+size.x/2, this.pos.y+size.y/2, target.pos.x+target.size.x/2, target.pos.y+target.size.y/2); } }
Questions and Final:
For this one, I thought it was better to have a bouncy still image, as it was the only one that was completely dead. I used the same class for both as they had the same charactaristics.
Sprites:
Questions:
Final:
Code:
class Question extends Creature { SoundFile sound; Question(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames, PApplet sketch) { super(x, y, r, g, _img, w, h, num_frames); speed.x=0; speed.y=-2; sound = new SoundFile(sketch, "token.mp3"); } void update() { gravity(); if (speed.y==0) { speed.y=-2; } pos.x +=speed.x; pos.y +=speed.y; } float distance(Player target) { return dist(this.pos.x, this.pos.y, target.pos.x, target.pos.y); } }
Game:
For the full game, I made a game class that allows me to create a game object whenever I want to start the game. This helps with restarting and waiting for the user to press a key to start; as I just create a game object if the player is dead and the user presses enter at the welcom screen.
My game class only had the creation of all the game objects and the display call for them.
Code:
class Game { // int ground = 100; public int score = 0; boolean won; Player myplayer; Platforms[] plats = new Platforms[5]; Platforms groundd; int platLocH[] = new int[5]; int platLocW[] = new int[5]; Monster[] myMonsters = new Monster[6]; Question[] myQuestions = new Question[5]; Question myfinal; Game(PApplet sketch, PImage playerImg, PImage[] monsters, PImage questionIMG, PImage finalIMG) { // creating ground groundd = new Platforms(0, heightt-ground, widthh, ground, 0); // creating platforms for (int i=0; i<plats.length; i++) { platLocH[i] = (heightt-255)-i*155; platLocW[i] = int(random(0, widthh-500)); plats[i]= new Platforms(platLocW[i], platLocH[i], 600, heightt/25, 15); } // creating Monsters(1@ground & 4 at plats 0-3) int n; float m; for (int i=4; i>-1; i--) { n = int(random(0, 3)); myMonsters[i]= new Monster(platLocW[i]+60, platLocH[i]-60*(monsters[n].width/monsters[n].height)-10, 60, ground, monsters[n], monsters[n].width, monsters[n].height, 2, platLocW[i]+random(40, 50), platLocW[i]+random(160, 220), sketch); } n = int(random(0, 3)); m = random(widthh/2, widthh-450); myMonsters[5] = new Monster(m+60, heightt-ground, 60, ground, monsters[n], monsters[n].width, monsters[n].height, 2, m+random(40, 50), m+random(160, 220), sketch); // creating Questions for (int i=3; i>-1; i--) { myQuestions[i]=new Question(platLocW[i]+400, platLocH[i]-60*(questionIMG.width/questionIMG.height)-50, 60, ground, questionIMG, questionIMG.width, questionIMG.height, 1, sketch); } myQuestions[4]=new Question(m+400, heightt-ground, 60, ground, questionIMG, questionIMG.width, questionIMG.height, 1, sketch); // creating final myfinal = new Question(platLocW[4]+400, platLocH[4]-80*(finalIMG.width/finalIMG.height)-50, 80, ground, finalIMG, finalIMG.width, finalIMG.height, 1, sketch); myfinal.alive=false; // creating Player myplayer = new Player(20, height-ground-180, 70, ground, playerImg, playerImg.width, playerImg.height, 9, sketch); won = false; } void main() { for (int i = 0; i<5; i++) { plats[i].display(); if (myQuestions[i].alive) { myQuestions[i].display(); } } groundd.display(); myplayer.display(); for (int i=0; i<6; i++) { if (myMonsters[i].alive) { myMonsters[i].display(); } } if (score == myQuestions.length) { myfinal.alive = true; myfinal.display(); } } }
Start and end screens and Key presses:
I made functions to display text at the start and end of each game. This allowed me to easily call either whenever needed.
I reset my game variables in the end screen functions, but that is just to avoid unexpected errors.
void startSc() { textFont(f, 80); fill(255); textAlign(CENTER); text("Dead Student", widthh/2, heightt/2-100); textFont(f, 40); text("press enter to start", widthh/2, heightt/2-10); textFont(f, 20); text("Collect the questions to get to final paper", widthh/2, heightt/2+100); text("You can kill monsters by jumping on them", widthh/2, heightt/2+130); text("Please use left, right, and up arrows to play", widthh/2, heightt/2+190); } void endSc() { if (game.won) { textFont(f, 40); fill(255); textAlign(CENTER); text("YOU WON!!", widthh/2, heightt/2); text("YOUR GRADE IS:", widthh/2, heightt/2+50); text(String.valueOf(game.score)+" /10", widthh/2, heightt/2+100); text("CONGRATS!!", widthh/2, heightt/2+150); textFont(f, 20); text("press r to reset", widthh/2, heightt/2+200); } else { for (int i=0; i<game.myMonsters.length; i++) { game.myMonsters[i].alive=true; } for (int i=0; i<game.myQuestions.length; i++) { game.myQuestions[i].alive=true; } textFont(f, 40); fill(255); textAlign(CENTER); text("YOU DIED", widthh/2, heightt/2); text("YOUR GRADE IS:", widthh/2, heightt/2+50); text(String.valueOf(game.score)+" /10", widthh/2, heightt/2+100); textFont(f, 20); text("press r to reset", widthh/2, heightt/2+150); } }
Key Pressed/Released Code:
I created the game when the user presses enter;
void keyPressed() { if (keyCode == 10 && started==false) {//enter started = true; game = new Game(this, playerimg, monsters, question, finall); } else if (keyCode==82 && started==true) {//r-> reset started=false; startSc(); } else if (started==true && game.won==false) { if (keyCode == LEFT) { game.myplayer.left = true; } if (keyCode==RIGHT) { game.myplayer.right = true; } if (keyCode==UP) { game.myplayer.up = true; } } } void keyReleased() { if (started==true) { if (keyCode == LEFT) { game.myplayer.left = false; } if (keyCode==RIGHT) { game.myplayer.right = false; } if (keyCode==UP) { game.myplayer.up = false; game.myplayer.secondJump=true; } } }
Pictures:
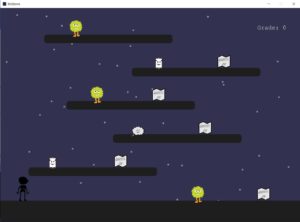
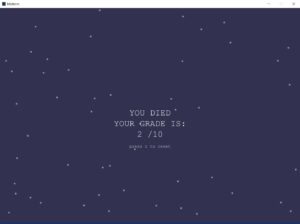
Video:
Google Drive Folder:
https://drive.google.com/drive/folders/1cQJcHDHnX_z_WFUf1K8c1MLbl8bZIeUl?usp=sharing
Full Code:
import processing.sound.*; int heightt= 1000; int widthh = 1400; PFont f; class Platforms { PVector pos; //mid point float pHeight; float pWidth; float widths; float rad; Platforms(float x, float y, float w, float h, float r) { pos = new PVector(x, y); pHeight = h; pWidth = w; rad = r; } void display() { noStroke(); rectMode(CORNER); fill(30); rect(pos.x, pos.y, pWidth, pHeight, rad); } } class Bg { float[] x = new float[50]; float[] y = new float[50]; Bg() { for (int i=0; i<50; i++) { x[i] = random(0, widthh); y[i] = random(0, heightt); } } void drawBG() { if (frameCount%50==0) { for (int i=0; i<50; i++) { x[i] = random(0, width); y[i] = random(0, height); } } background(50, 50, 80); for (int i=0; i<50; i++) { for (int j=0; j<10; j++) { noStroke(); fill(255, 25); ellipse(x[i], y[i], 1+j, 1+j); } } } } public class Creature { // Attributes PVector pos; PVector speed; boolean alive; float ground; PVector size; PVector imgSize; int numFrames; PImage imgs; PImage[] img; int frame; boolean dir; //left = 0 right = 1 boolean secondJump = false; public Creature(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames) { pos= new PVector(x, y); speed= new PVector(0, 0); alive = true; ground = height-g; numFrames = num_frames; imgSize = new PVector(_img.width/num_frames, _img.height); size = new PVector (r, imgSize.y*(r/imgSize.x)); imgs = _img; img = new PImage[numFrames]; for (int i=0; i<numFrames; i++) { img[i] = imgs.get( int((imgSize.x)*i), 0, int(imgSize.x), int(imgSize.y)); } // for (int y=0; y < 4; y++) { // for (int x=0; x< 12; x++) { // sprites[y][x] = spritesheet.get(x*w, y*h, w, h); // } // } frame = 0; dir = true; } void gravity() { if (pos.y<ground-size.y+10 ||pos.y<ground-size.y ) { speed.y+=0.2; } else { speed.y=0; pos.y = constrain(pos.y, ground-size.y, ground-size.y+10); secondJump=false; } for (int p=game.plats.length-1; p>=0; p--) { if ((pos.y+size.y <=game.plats[p].pos.y+15)//height && pos.x+size.x/3>=game.plats[p].pos.x//right && pos.x-size.x/3 <= game.plats[p].pos.x+game.plats[p].pWidth) {//left ground = game.plats[p].pos.y; break; } else { ground = heightt-game.ground; } } } void update() { gravity(); pos.x += speed.x; pos.y += speed.y; } void display() { update(); // println(this.getClass().getName()); if (dir && alive) { scale(1, 1); image(img[frame], pos.x, pos.y, size.x, size.y); } else if (!dir && alive) { if (this.getClass().getName()=="Midterm$Player") { pushMatrix(); scale(-1, 1); image(img[frame], -pos.x, pos.y, size.x, size.y); popMatrix(); } else if (this.getClass().getName()=="Midterm$Monster") { image(img[frame], pos.x, pos.y, size.x, size.y); } } } } class Player extends Creature { boolean left; boolean up; boolean right; SoundFile jump; int count; Player(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames, PApplet sketch) { super(x, y, r, g, _img, w, h, num_frames); left = false; up = false; right = false; jump = new SoundFile(sketch, "jump.wav"); count = frameCount; } void update() { gravity(); //==================== if (left==true) { speed.x=-3; dir=false; } else if (right==true) { speed.x=3; dir=true; } else { speed.x=0; } //==================== if (up==true && pos.y>=ground-size.y &&secondJump==false) { jump.play(); speed.y = -6; } else if (up==true && speed.y<0 && secondJump==true && pos.y<ground-size.y) { jump.play(); speed.y = -8; secondJump=false; } pos.x +=speed.x; pos.y +=speed.y; if (frameCount%5 == 0 && speed.x !=0 && speed.y ==0) { frame= (frame+1)%numFrames; } else if (speed.x==0) { frame=8; } //==================== if (pos.x<=0) { pos.x = 0; } //==================== if (pos.x+size.x>= widthh) { pos.x = widthh- size.x; } else if (pos.x<size.x) { pos.x = size.x; } //==================== for (int i =0; i<game.myMonsters.length; i++) { float numME = pow((pow(size.x, 2)+(pow(size.y, 2))), 0.5); float numthem = pow((pow(game.myMonsters[i].size.x, 2)+(pow(game.myMonsters[i].size.y, 2))), 0.5); if (game.myMonsters[i].distance(this)<=numME/2.5+numthem/2.5 && game.myMonsters[i].alive) { if (speed.y>0) { game.myMonsters[i].alive=false; game.myMonsters[i].sound2.play(); } else { game.myMonsters[i].sound1.play(); alive = false; } } } for (int i =0; i<game.myQuestions.length; i++) { if (game.myQuestions[i].distance(this)<=size.x/2+game.myQuestions[i].size.x/2 && game.myQuestions[i].alive) { game.score++; game.myQuestions[i].sound.play(); game.myQuestions[i].alive = false; } } if (game.myfinal.distance(this)<=size.x/2+game.myfinal.size.x/2 && game.myfinal.alive) { game.score+=5; game.myfinal.sound.play(); game.myfinal.alive = false; game.won= true; } } } class Monster extends Creature { SoundFile sound1, sound2; float xleft, xright; Monster(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames, float x_left, float x_right, PApplet sketch) { super(x, y, r, g, _img, w, h, num_frames); speed.x=random(0.3,0.9); speed.y=0; xleft = x_left; xright = x_right; sound1 = new SoundFile(sketch, "eat.wav"); sound2 = new SoundFile(sketch, "kill.wav"); } void update() { gravity(); if (pos.x<=xleft) { dir = true; frame= (frame+1)%numFrames; speed.x*=-1; } else if (pos.x>=xright) { dir = false; frame= (frame+1)%numFrames; speed.x*=-1; } pos.x +=speed.x; pos.y +=speed.y; } float distance(Player target) { return dist(this.pos.x+size.x/2, this.pos.y+size.y/2, target.pos.x+target.size.x/2, target.pos.y+target.size.y/2); } } class Question extends Creature { SoundFile sound; Question(float x, float y, float r, float g, PImage _img, float w, float h, int num_frames, PApplet sketch) { super(x, y, r, g, _img, w, h, num_frames); speed.x=0; speed.y=-2; sound = new SoundFile(sketch, "token.mp3"); } void update() { gravity(); if (speed.y==0) { speed.y=-2; } pos.x +=speed.x; pos.y +=speed.y; } float distance(Player target) { return dist(this.pos.x, this.pos.y, target.pos.x, target.pos.y); } } class Game { // int ground = 100; public int score = 0; boolean won; Player myplayer; Platforms[] plats = new Platforms[5]; Platforms groundd; int platLocH[] = new int[5]; int platLocW[] = new int[5]; Monster[] myMonsters = new Monster[6]; Question[] myQuestions = new Question[5]; Question myfinal; Game(PApplet sketch, PImage playerImg, PImage[] monsters, PImage questionIMG, PImage finalIMG) { // creating ground groundd = new Platforms(0, heightt-ground, widthh, ground, 0); // creating platforms for (int i=0; i<plats.length; i++) { platLocH[i] = (heightt-255)-i*155; platLocW[i] = int(random(0, widthh-500)); plats[i]= new Platforms(platLocW[i], platLocH[i], 600, heightt/25, 15); } // creating Monsters(1@ground & 4 at plats 0-3) int n; float m; for (int i=4; i>-1; i--) { n = int(random(0, 3)); myMonsters[i]= new Monster(platLocW[i]+60, platLocH[i]-60*(monsters[n].width/monsters[n].height)-10, 60, ground, monsters[n], monsters[n].width, monsters[n].height, 2, platLocW[i]+random(40, 50), platLocW[i]+random(160, 220), sketch); } n = int(random(0, 3)); m = random(widthh/2, widthh-450); myMonsters[5] = new Monster(m+60, heightt-ground, 60, ground, monsters[n], monsters[n].width, monsters[n].height, 2, m+random(40, 50), m+random(160, 220), sketch); // creating Questions for (int i=3; i>-1; i--) { myQuestions[i]=new Question(platLocW[i]+400, platLocH[i]-60*(questionIMG.width/questionIMG.height)-50, 60, ground, questionIMG, questionIMG.width, questionIMG.height, 1, sketch); } myQuestions[4]=new Question(m+400, heightt-ground, 60, ground, questionIMG, questionIMG.width, questionIMG.height, 1, sketch); // creating final myfinal = new Question(platLocW[4]+400, platLocH[4]-80*(finalIMG.width/finalIMG.height)-50, 80, ground, finalIMG, finalIMG.width, finalIMG.height, 1, sketch); myfinal.alive=false; // creating Player myplayer = new Player(20, height-ground-180, 70, ground, playerImg, playerImg.width, playerImg.height, 9, sketch); won = false; } void main() { for (int i = 0; i<5; i++) { plats[i].display(); if (myQuestions[i].alive) { myQuestions[i].display(); } } groundd.display(); myplayer.display(); for (int i=0; i<6; i++) { if (myMonsters[i].alive) { myMonsters[i].display(); } } if (score == myQuestions.length) { myfinal.alive = true; myfinal.display(); } } } void startSc() { textFont(f, 80); fill(255); textAlign(CENTER); text("Dead Student", widthh/2, heightt/2-100); textFont(f, 40); text("press enter to start", widthh/2, heightt/2-10); textFont(f, 20); text("Collect the questions to get to final paper", widthh/2, heightt/2+100); text("You can kill monsters by jumping on them", widthh/2, heightt/2+130); text("Please use left, right, and up arrows to play", widthh/2, heightt/2+190); } void endSc() { if (game.won) { textFont(f, 40); fill(255); textAlign(CENTER); text("YOU WON!!", widthh/2, heightt/2); text("YOUR GRADE IS:", widthh/2, heightt/2+50); text(String.valueOf(game.score)+" /10", widthh/2, heightt/2+100); text("CONGRATS!!", widthh/2, heightt/2+150); textFont(f, 20); text("press r to reset", widthh/2, heightt/2+200); } else { for (int i=0; i<game.myMonsters.length; i++) { game.myMonsters[i].alive=true; } for (int i=0; i<game.myQuestions.length; i++) { game.myQuestions[i].alive=true; } textFont(f, 40); fill(255); textAlign(CENTER); text("YOU DIED", widthh/2, heightt/2); text("YOUR GRADE IS:", widthh/2, heightt/2+50); text(String.valueOf(game.score)+" /10", widthh/2, heightt/2+100); textFont(f, 20); text("press r to reset", widthh/2, heightt/2+150); } } Game game; PImage playerimg; PImage[] monsters = new PImage[3]; PImage question, finall, bgImg; Bg bckgrnd; boolean started; SoundFile backgroundSound; void loadStuff() { playerimg = loadImage("playerog.png"); monsters[0] = loadImage("phone.png"); monsters[1] = loadImage("anxiety.png"); monsters[2] = loadImage("thoughts.png"); question = loadImage("question.png"); finall = loadImage("final.png"); bgImg = loadImage("cloud.png"); backgroundSound = new SoundFile(this, "bg.wav"); } void setup() { size(1400, 1000); loadStuff(); f = createFont("Courier New", 32); started = false; bckgrnd = new Bg(); } void draw() { background(100); bckgrnd.drawBG(); if (!backgroundSound.isPlaying()) { backgroundSound.play(); } if (started) { if (game.myplayer.alive && game.won==false) { game.main(); textFont(f, 28); fill(255); textAlign(LEFT); text("Grade: "+String.valueOf(game.score), widthh-200, 100); } else { endSc(); } } else { startSc(); } } void keyPressed() { if (keyCode == 10 && started==false) {//enter started = true; game = new Game(this, playerimg, monsters, question, finall); } else if (keyCode==82 && started==true) {//r-> reset started=false; startSc(); } else if (started==true && game.won==false) { if (keyCode == LEFT) { game.myplayer.left = true; } if (keyCode==RIGHT) { game.myplayer.right = true; } if (keyCode==UP) { game.myplayer.up = true; } } } void keyReleased() { if (started==true) { if (keyCode == LEFT) { game.myplayer.left = false; } if (keyCode==RIGHT) { game.myplayer.right = false; } if (keyCode==UP) { game.myplayer.up = false; game.myplayer.secondJump=true; } } }