As part of the midterm project, I am designing a game around the theme of coping with stress as an NYUAD student. It was really difficult for me to settle on one single idea as I found myself trying several different things for hours and then ultimately deciding on this idea finally a night before the submission is due. The intended game design is to collect the falling activities (that increase or help cope with stress levels). Each activity is associated with a certain number of points and special abilities such as boosted/ slowed down the speed of the player. The player moves across the screen horizontally and tries to catch points. All missed points are added to the stress level and if the stress level reaches 320, the player loses. If the player collects 320 points first, he/she wins. The user is allowed the option to restart the game also.
Production
To start with the implementation, I sketched out some layouts of my instruction screen and main page.
I then created a simplistic version of these pages to later come back and design them. The whole navigation across the game is based on keypresses and mouse presses. Specific keys are set to allow the selection in the game and proceed forward with the setting. Below are the snapshots:
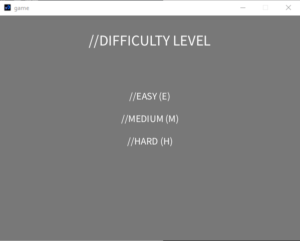
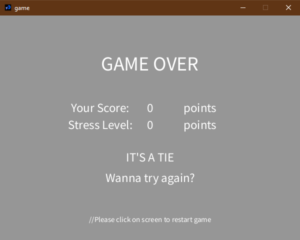
Here is the code worked on until now
//Global variables responsible for various states in game
//That includes: difficulty select, playing game, game over
String difficulty = "EASY";
Activity[] activityarray;
start = new Game(state, difficulty);
activityarray = new Activity[30];
//choose random x values and drop activities
for(int i=0; i<activityarray.length; i++){
float x_pos = random(0,width-50);
float type = random(1,50);
String activity_ability = "FOOD";
//randomly choosing ability
activity_ability = "LEISURE";
activity_ability = "GOODGRADES";
if(type > 10 && type <=25){
activity_ability = "DEADLINE";
//create new activity object
activityarray[i] = Activity(x_pos,start.speed,activity_ability);
Game(String state, String difficulty){
textAlign(CENTER,CENTER);
if(lostpoints >= 320 || score >= 320){
//load the main landing page
for(int y=0;y<height;y++){
for(int x=0;x<width; x++){
pixels[index] = color(map(y,0,height,155,0),0,map(y,0,height,255,0),50);
text("//GAME NAME",width/2,height/3);
text("START GAME (G)",width/2,2*height/3 - height/14);
text("INSTRUCTIONS (I)",width/2,2*height/3);
text("Press corresponding keys to initiate the game",width/2,height-height/14);
//loading the instructions page
if(mode=="INSTRUCTIONS"){
text("//INSTRUCTIONS",width/2,height/10);
text("//Use arrow keys to move across the screen",width/2,2.5*height/10);
text("//Eat healthy to gain +10 pts",width/2,3.5*height/10);
text("//Perform well on assignment to get +20 pts ",width/2,4.5*height/10);
text("//Do refresing leisure activities to get boosted speed",width/2,5.5*height/10);
text("//Deadline pressure decreases speed",width/2,6.5*height/10);
text("//Missed pts are added to stress level. First to reach 320 pts win",width/2,7.5*height/10);
text("//START GAME (G)",width/2,9*height/10);
text("//DIFFICULTY LEVEL",width/2,height/10);
text("//EASY (E)",width/2,3.5*height/10);
text("//MEDIUM (M)",width/2,4.5*height/10);
text("//HARD (H)",width/2,5.5*height/10);
text("GAME OVER",width/2, height/5);
text("Your Score:",width/2 - width/6, height/3 +height/15);
text("Stress Level:",width/2 -width/6, height/3 + height/7);
text(score,width/2, height/3 +height/15);
text(lostpoints,width/2, height/3 + height/7);
text("points",width/2 + width/6, height/3 +height/15);
text("points",width/2 +width/6, height/3 + height/7);
text("IT'S A TIE\nWanna try again?", width/2, 2*height/3);
text("YOU WON", width/2, 2*height/3);
text("YOU LOST\nWanna try again?", width/2, 2*height/3);
text("//Please click on screen to restart game",width/2,9*height/10);
float xPos = width/2 - pwidth/2;
float yPos = height - (height - base) - pheight;
//tracking the time when boosted speed
//tracking the time when slowed speed
//update the position on screen
//change color based on ability
ellipse(xPos,yPos,pwidth,pheight);
//update the position of the player
if(left==true && xPos >=0){
if(right==true && xPos <= width - pwidth){
//Class of falling activities/ points
Activity(float xpos, float s, String a){
if(ability == "GOODGRADES"){
activityimg = loadImage("test.png");
else if(ability == "LEISURE"){
activityimg = loadImage("leisure.png");
else if(ability == "DEADLINE"){
activityimg = loadImage("deadline.png");
activityimg = loadImage("food.png");
image(activityimg,xloc,yloc,awidth,aheight);
if((player.xPos + player.pwidth >= xloc) && (player.xPos <= xloc + awidth)){
if((yloc + aheight >= player.yPos) && (yloc <= player.pheight + player.yPos)){
//check if it collides with special activity
if(ability == "LEISURE"){
if(ability == "DEADLINE"){
//keep track of key presses on screen
if(start.mode == "MAIN"){
if(keyCode == 73){ //73 = 'i'
start.mode = "INSTRUCTIONS";
if(keyCode == 71){ //71 = 'g'
start.mode = "DIFFICULTY";
if(start.mode == "INSTRUCTIONS"){
if(keyCode == 71){ //71 = 'g'
start.mode = "DIFFICULTY";
if(start.mode == "DIFFICULTY"){
if(keyCode == 69){ //71 = 'e'
if(keyCode == 77){ //71 = 'm'
if(keyCode == 72){ //71 = 'h'
//stop motion when key is released
if(start.mode == "PLAY"){
start = new Game("MAIN","EASY");
//Global variables responsible for various states in game
//That includes: difficulty select, playing game, game over
Game start;
Player player;
String state = "MAIN";
String difficulty = "EASY";
Activity[] activityarray;
//setup the game
void setup(){
size(600,450);
start = new Game(state, difficulty);
activityarray = new Activity[30];
//choose random x values and drop activities
for(int i=0; i<activityarray.length; i++){
float x_pos = random(0,width-50);
float type = random(1,50);
String activity_ability = "FOOD";
//randomly choosing ability
if(type < 5){
activity_ability = "LEISURE";
}
else if(type > 45){
activity_ability = "GOODGRADES";
}
if(type > 10 && type <=25){
activity_ability = "DEADLINE";
}
//create new activity object
activityarray[i] = Activity(x_pos,start.speed,activity_ability);
}
}
//game class
class Game{
String mode;
String level;
int score = 0;
int lostpoints = 0;
float speed = 0;
float drop_speed = 30;
float frames = 0;
Game(String state, String difficulty){
mode = state;
level = difficulty;
}
void display(){
textAlign(CENTER,CENTER);
//check for game over
if(lostpoints >= 320 || score >= 320){
mode = "OVER";
}
//load the main landing page
if(mode=="MAIN"){
loadPixels();
for(int y=0;y<height;y++){
for(int x=0;x<width; x++){
int index = x+y*width;
pixels[index] = color(map(y,0,height,155,0),0,map(y,0,height,255,0),50);
}
}
updatePixels();
textSize(50);
text("//GAME NAME",width/2,height/3);
textSize(18);
text("START GAME (G)",width/2,2*height/3 - height/14);
text("INSTRUCTIONS (I)",width/2,2*height/3);
textSize(14);
text("Press corresponding keys to initiate the game",width/2,height-height/14);
}
//loading the instructions page
if(mode=="INSTRUCTIONS"){
background(120);
textSize(30);
text("//INSTRUCTIONS",width/2,height/10);
textSize(15);
text("//Use arrow keys to move across the screen",width/2,2.5*height/10);
text("//Eat healthy to gain +10 pts",width/2,3.5*height/10);
text("//Perform well on assignment to get +20 pts ",width/2,4.5*height/10);
text("//Do refresing leisure activities to get boosted speed",width/2,5.5*height/10);
text("//Deadline pressure decreases speed",width/2,6.5*height/10);
text("//Missed pts are added to stress level. First to reach 320 pts win",width/2,7.5*height/10);
textSize(22);
text("//START GAME (G)",width/2,9*height/10);
}
if(mode=="DIFFICULTY"){
background(120);
textSize(30);
text("//DIFFICULTY LEVEL",width/2,height/10);
textSize(20);
text("//EASY (E)",width/2,3.5*height/10);
text("//MEDIUM (M)",width/2,4.5*height/10);
text("//HARD (H)",width/2,5.5*height/10);
}
//game over screen
if(mode=="OVER"){
background(150);
textSize(40);
text("GAME OVER",width/2, height/5);
textSize(25);
text("Your Score:",width/2 - width/6, height/3 +height/15);
text("Stress Level:",width/2 -width/6, height/3 + height/7);
text(score,width/2, height/3 +height/15);
text(lostpoints,width/2, height/3 + height/7);
text("points",width/2 + width/6, height/3 +height/15);
text("points",width/2 +width/6, height/3 + height/7);
if(score>=lostpoints){
if(score==lostpoints){
text("IT'S A TIE\nWanna try again?", width/2, 2*height/3);
}
else{
text("YOU WON", width/2, 2*height/3);
}
}
else{
text("YOU LOST\nWanna try again?", width/2, 2*height/3);
}
textSize(15);
text("//Please click on screen to restart game",width/2,9*height/10);
}
//main game screen
if(mode=="PLAY"){
background(255);
}
}
}
//player class
class Player{
float pwidth= 100;
float pheight = 100;
float base = 600;
float xPos = width/2 - pwidth/2;
float yPos = height - (height - base) - pheight;
boolean left = false;
boolean right = false;
float speed = 5;
float fast_time = 0;
float slow_time = 0;
Player(){}
void display(){
//tracking the time when boosted speed
if(speed == 10){
fast_time += 1;
//last 100 frames
if(fast_time == 100){
fast_time = 0;
speed = 5;
}
}
//tracking the time when slowed speed
if(speed == 1){
fast_time += 1;
//last 100 frames
if(fast_time == 100){
fast_time = 0;
speed = 5;
}
}
//update the position on screen
update();
//change color based on ability
if(speed == 1){
tint(214,87,77);
}
else if(speed == 10){
tint(237,211,81);
}
//draw the player
fill(50,0,50);
ellipse(xPos,yPos,pwidth,pheight);
//stop tint
noTint();
}
//update the position of the player
void update(){
if(left==true && xPos >=0){
xPos -= speed;
}
if(right==true && xPos <= width - pwidth){
xPos += speed;
}
}
}
//Class of falling activities/ points
class Activity{
//resizing required
float awidth = 50;
float aheight = 40;
//coordinates
float yloc = -aheight;
float xloc;
float speed;
String ability;
//standard point
int point = 10;
//image
PImage activityimg;
Activity(float xpos, float s, String a){
xloc = xpos;
speed = s;
ability = a;
//updating point values
if(ability == "GOODGRADES"){
activityimg = loadImage("test.png");
point = 20;
}
else if(ability == "LEISURE"){
activityimg = loadImage("leisure.png");
}
else if(ability == "DEADLINE"){
point = 0;
activityimg = loadImage("deadline.png");
}
else{
activityimg = loadImage("food.png");
}
}
//display the images
void display(){
image(activityimg,xloc,yloc,awidth,aheight);
}
//update the locations
void update(){
//move down
yloc += speed;
}
//check for collisions
boolean collisions(){
if((player.xPos + player.pwidth >= xloc) && (player.xPos <= xloc + awidth)){
if((yloc + aheight >= player.yPos) && (yloc <= player.pheight + player.yPos)){
//check if it collides with special activity
if(ability == "LEISURE"){
player.speed = 10;
}
if(ability == "DEADLINE"){
player.speed = 1;
}
return true;
}
}
return false;
}
}
void draw(){
start.display();
}
//keep track of key presses on screen
void keyPressed(){
if(start.mode == "MAIN"){
if(keyCode == 73){ //73 = 'i'
start.mode = "INSTRUCTIONS";
}
if(keyCode == 71){ //71 = 'g'
start.mode = "DIFFICULTY";
}
}
if(start.mode == "INSTRUCTIONS"){
if(keyCode == 71){ //71 = 'g'
start.mode = "DIFFICULTY";
}
}
if(start.mode == "DIFFICULTY"){
if(keyCode == 69){ //71 = 'e'
difficulty = "EASY";
start.speed = 3;
start.mode = "PLAY";
}
if(keyCode == 77){ //71 = 'm'
difficulty = "MEDIUM";
start.speed = 6;
start.mode = "PLAY";
}
if(keyCode == 72){ //71 = 'h'
difficulty = "HARD";
start.speed = 10;
start.mode = "PLAY";
}
}
//move until key pressed
if(start.mode=="PLAY"){
if(keyCode == RIGHT){
player.right = true;
}
if(keyCode == LEFT){
player.left = true;
}
}
}
//stop motion when key is released
void keyReleased(){
if(start.mode == "PLAY"){
if(keyCode == RIGHT){
player.right = false;
}
if(keyCode == LEFT){
player.left = false;
}
}
}
//replay the game
void mouseClicked(){
if(start.mode=="OVER"){
start.mode = "MAIN";
noTint();
start = new Game("MAIN","EASY");
}
}
//Global variables responsible for various states in game
//That includes: difficulty select, playing game, game over
Game start;
Player player;
String state = "MAIN";
String difficulty = "EASY";
Activity[] activityarray;
//setup the game
void setup(){
size(600,450);
start = new Game(state, difficulty);
activityarray = new Activity[30];
//choose random x values and drop activities
for(int i=0; i<activityarray.length; i++){
float x_pos = random(0,width-50);
float type = random(1,50);
String activity_ability = "FOOD";
//randomly choosing ability
if(type < 5){
activity_ability = "LEISURE";
}
else if(type > 45){
activity_ability = "GOODGRADES";
}
if(type > 10 && type <=25){
activity_ability = "DEADLINE";
}
//create new activity object
activityarray[i] = Activity(x_pos,start.speed,activity_ability);
}
}
//game class
class Game{
String mode;
String level;
int score = 0;
int lostpoints = 0;
float speed = 0;
float drop_speed = 30;
float frames = 0;
Game(String state, String difficulty){
mode = state;
level = difficulty;
}
void display(){
textAlign(CENTER,CENTER);
//check for game over
if(lostpoints >= 320 || score >= 320){
mode = "OVER";
}
//load the main landing page
if(mode=="MAIN"){
loadPixels();
for(int y=0;y<height;y++){
for(int x=0;x<width; x++){
int index = x+y*width;
pixels[index] = color(map(y,0,height,155,0),0,map(y,0,height,255,0),50);
}
}
updatePixels();
textSize(50);
text("//GAME NAME",width/2,height/3);
textSize(18);
text("START GAME (G)",width/2,2*height/3 - height/14);
text("INSTRUCTIONS (I)",width/2,2*height/3);
textSize(14);
text("Press corresponding keys to initiate the game",width/2,height-height/14);
}
//loading the instructions page
if(mode=="INSTRUCTIONS"){
background(120);
textSize(30);
text("//INSTRUCTIONS",width/2,height/10);
textSize(15);
text("//Use arrow keys to move across the screen",width/2,2.5*height/10);
text("//Eat healthy to gain +10 pts",width/2,3.5*height/10);
text("//Perform well on assignment to get +20 pts ",width/2,4.5*height/10);
text("//Do refresing leisure activities to get boosted speed",width/2,5.5*height/10);
text("//Deadline pressure decreases speed",width/2,6.5*height/10);
text("//Missed pts are added to stress level. First to reach 320 pts win",width/2,7.5*height/10);
textSize(22);
text("//START GAME (G)",width/2,9*height/10);
}
if(mode=="DIFFICULTY"){
background(120);
textSize(30);
text("//DIFFICULTY LEVEL",width/2,height/10);
textSize(20);
text("//EASY (E)",width/2,3.5*height/10);
text("//MEDIUM (M)",width/2,4.5*height/10);
text("//HARD (H)",width/2,5.5*height/10);
}
//game over screen
if(mode=="OVER"){
background(150);
textSize(40);
text("GAME OVER",width/2, height/5);
textSize(25);
text("Your Score:",width/2 - width/6, height/3 +height/15);
text("Stress Level:",width/2 -width/6, height/3 + height/7);
text(score,width/2, height/3 +height/15);
text(lostpoints,width/2, height/3 + height/7);
text("points",width/2 + width/6, height/3 +height/15);
text("points",width/2 +width/6, height/3 + height/7);
if(score>=lostpoints){
if(score==lostpoints){
text("IT'S A TIE\nWanna try again?", width/2, 2*height/3);
}
else{
text("YOU WON", width/2, 2*height/3);
}
}
else{
text("YOU LOST\nWanna try again?", width/2, 2*height/3);
}
textSize(15);
text("//Please click on screen to restart game",width/2,9*height/10);
}
//main game screen
if(mode=="PLAY"){
background(255);
}
}
}
//player class
class Player{
float pwidth= 100;
float pheight = 100;
float base = 600;
float xPos = width/2 - pwidth/2;
float yPos = height - (height - base) - pheight;
boolean left = false;
boolean right = false;
float speed = 5;
float fast_time = 0;
float slow_time = 0;
Player(){}
void display(){
//tracking the time when boosted speed
if(speed == 10){
fast_time += 1;
//last 100 frames
if(fast_time == 100){
fast_time = 0;
speed = 5;
}
}
//tracking the time when slowed speed
if(speed == 1){
fast_time += 1;
//last 100 frames
if(fast_time == 100){
fast_time = 0;
speed = 5;
}
}
//update the position on screen
update();
//change color based on ability
if(speed == 1){
tint(214,87,77);
}
else if(speed == 10){
tint(237,211,81);
}
//draw the player
fill(50,0,50);
ellipse(xPos,yPos,pwidth,pheight);
//stop tint
noTint();
}
//update the position of the player
void update(){
if(left==true && xPos >=0){
xPos -= speed;
}
if(right==true && xPos <= width - pwidth){
xPos += speed;
}
}
}
//Class of falling activities/ points
class Activity{
//resizing required
float awidth = 50;
float aheight = 40;
//coordinates
float yloc = -aheight;
float xloc;
float speed;
String ability;
//standard point
int point = 10;
//image
PImage activityimg;
Activity(float xpos, float s, String a){
xloc = xpos;
speed = s;
ability = a;
//updating point values
if(ability == "GOODGRADES"){
activityimg = loadImage("test.png");
point = 20;
}
else if(ability == "LEISURE"){
activityimg = loadImage("leisure.png");
}
else if(ability == "DEADLINE"){
point = 0;
activityimg = loadImage("deadline.png");
}
else{
activityimg = loadImage("food.png");
}
}
//display the images
void display(){
image(activityimg,xloc,yloc,awidth,aheight);
}
//update the locations
void update(){
//move down
yloc += speed;
}
//check for collisions
boolean collisions(){
if((player.xPos + player.pwidth >= xloc) && (player.xPos <= xloc + awidth)){
if((yloc + aheight >= player.yPos) && (yloc <= player.pheight + player.yPos)){
//check if it collides with special activity
if(ability == "LEISURE"){
player.speed = 10;
}
if(ability == "DEADLINE"){
player.speed = 1;
}
return true;
}
}
return false;
}
}
void draw(){
start.display();
}
//keep track of key presses on screen
void keyPressed(){
if(start.mode == "MAIN"){
if(keyCode == 73){ //73 = 'i'
start.mode = "INSTRUCTIONS";
}
if(keyCode == 71){ //71 = 'g'
start.mode = "DIFFICULTY";
}
}
if(start.mode == "INSTRUCTIONS"){
if(keyCode == 71){ //71 = 'g'
start.mode = "DIFFICULTY";
}
}
if(start.mode == "DIFFICULTY"){
if(keyCode == 69){ //71 = 'e'
difficulty = "EASY";
start.speed = 3;
start.mode = "PLAY";
}
if(keyCode == 77){ //71 = 'm'
difficulty = "MEDIUM";
start.speed = 6;
start.mode = "PLAY";
}
if(keyCode == 72){ //71 = 'h'
difficulty = "HARD";
start.speed = 10;
start.mode = "PLAY";
}
}
//move until key pressed
if(start.mode=="PLAY"){
if(keyCode == RIGHT){
player.right = true;
}
if(keyCode == LEFT){
player.left = true;
}
}
}
//stop motion when key is released
void keyReleased(){
if(start.mode == "PLAY"){
if(keyCode == RIGHT){
player.right = false;
}
if(keyCode == LEFT){
player.left = false;
}
}
}
//replay the game
void mouseClicked(){
if(start.mode=="OVER"){
start.mode = "MAIN";
noTint();
start = new Game("MAIN","EASY");
}
}
Challenges
Aside from taking so long to decide on one single project, I have encountered several issues. First and foremost, my whole game can not be executed once all the classes have been defined and all the code has been linked together. I have done my best to make as much progress I could on the overall game, but I am hoping with a few more hours of work, I’ll be able to finish off linking my code and making it work.
More to Add
Firstly, I need to finish off the basic code and allow for the basic functioning of the game to be complete. Then, I need to work on the design and aesthetics of the screen and overall game. Lastly, I need to resize my images and add sound to the game.
Sounds like a good game. the problem with you code not compiling, and the thing you asked be about the other day, is that when you are making your activity objects you don’t use the word new. Do it like this in line 30 with the word new included: activityarray[i] = new Activity(x_pos,start.speed,activity_ability); and then it will work. I think what I told you the other day is still a good thing to include though, only load the images once globally and then reuse them in you activity class.