I have a deep fascination for the Butterfly Effect so of course when it was mentioned in class, I wanted to take my assignment’s concept from it.
I started off with very high expectations. My idea was that I wanted something to represent the universe, and a butterfly flapping in front of it. But I quickly found out that to recreate a galaxy or something similar, I would pretty much have to copy reference code from somewhere.
I started experimenting on my own, and thought that beziers and curves might be useful. Curves served my purpose well enough, but I could not control them to cover the whole height of the screen like I had wanted. So, I settled on lines.
Now, I wanted to use noise to make it dynamic, but noise meant equal distances, and I wanted more chaos to represent the universe. So, I ended up with random. I had been drawing lines in the main sketch, but I realised they could be objects too. Here is my Line class. In another more complex version, it could have more functionality.
class Line{ float _x1; float _x2; color col; Line(){ _x1 = random(width); _x2 = random(width); col= color(random(100), random(100), random(255)); //more in the spectrum of blues to resemble galaxies } }
I also restricted the colour scale to a more blue-heavy tone because that reminds me of the universe. Here is the drawing with a static photo of the lines:
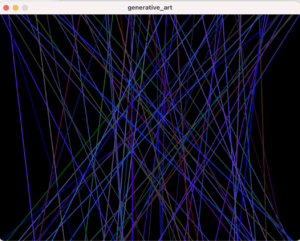
Then, I wanted the butterfly to be a class of its own too so that in variations, more butterfly objects could be inserted easily. In my vision for this drawing, I wanted it to be a white butterfly right in the middle of the screen. I used only ellipses, leaving out the “stick” of the body to make it look more stylistic. I used the translate(), rotate() and pushMatrix() and popMatrix() functionalities. The only thing left to figure out was the flapping itself, and I tried a couple of approaches. First I tried to change the rotation every few seconds (using frameCount) but that was looking too stilted and it didn’t look like flapping at all. I ended up just changing the heights and widths of the wings to give the illusion of movement. It’s still not perfect, but it’s better than what I thought was possible. My initial plan had also been that the flapping would change the noise and bring change on the screen, but since I used random I couldn’t do that.
Here is my Butterfly class:
class Butterfly{ int upper_width; //referring to the wings int lower_width; int upper_height; int lower_height; Butterfly(){ upper_width = 30; lower_width = 20; upper_height = 50; lower_height = 30; } void draw(){ //left wings pushMatrix(); translate(width/2, height/2); if(frameCount%50==0){ //for the occasional flapping effect upper_width = 20; upper_height = 60; lower_width = 10; lower_height = 40; } else { upper_width = 30; upper_height = 50; upper_width = 30; lower_width = 20; upper_height = 50; lower_height = 30; } rotate(PI*2-PI/4); noStroke(); fill(255); ellipse(-35, 19, lower_width, lower_height); ellipse(-20, 0, upper_width, upper_height); popMatrix(); //right wings pushMatrix(); translate(width/2, height/2); if(frameCount%50==0){ upper_width = 20; upper_height = 60; lower_width = 10; lower_height = 40; } else { upper_width = 30; upper_height = 50; upper_width = 30; lower_width = 20; upper_height = 50; lower_height = 30; } rotate(PI/4); noStroke(); fill(255); ellipse(35, 19, lower_width, lower_height); ellipse(20, 0, upper_width, upper_height); popMatrix(); } }
Here’s a comparison with one of my early trials with noise and the final version:
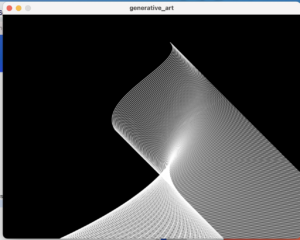
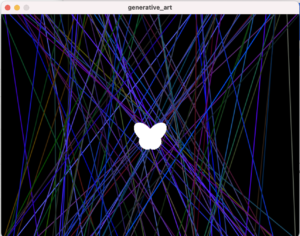
And here’s a video of the movement:
The main sketch ended up looking like:
int num_lines = int(random(75, 100)); Line[] lines = new Line[num_lines]; //array of Line objects Butterfly butterfly = new Butterfly(); //Butterfly object void setup(){ size(640, 480); } void draw(){ background(0); for(int i =0; i<num_lines; i++) { lines[i]=new Line(); strokeWeight(2); stroke(lines[i].col); line(lines[i]._x1, 0, lines[i]._x2, 480); //drawing top to bottom lines butterfly.draw(); //the butterfly with flapping effect will automatically be drawn on top } }
Overall, it ended up much different from the whimsical space-y theme I wanted, and seems more representative of the string theory or something in the background.
But, I guess that’s the Butterfly Effect for you.