I was quite excited for this week’s project as it was about making a game and thought of the ideas that I could pursue to make a game that would be fun to play. I was initially thinking of something where the user has a specific time limit to book the gym slot and if he is unable to do so, he loses the slot. But then I realized that the game does not implement the concept of classes that well and hence won’t be that relevant to this week’s topic. Therefore, I thought of making a shooter game in which you battle the world’s number one enemy, the Corona virus!
Difficulties:
- Although this game was really fun to make, I did run into a bunch of really annoying problems. The first major problem I ran into was to make bullets that would appear and shoot on my command. In my first attempt of doing so, I had created something in which when I would press the command to shoot, the bullet would just extend indefinitely and not work as a bullet does. After this was resolved, I faced another challenge of aligning the movement of the bullet with that of the player. Initially, when I would move the player, the bullet would not move accordingly and hence I needed to figure a way around that.
- The next major challenge that I faced was to actually find a game mechanic that would allow the bullet to kill the virus when it touched it. I was struggling to find a clean and simple solution to this problem as I was going with the incorrect approach of emphasizing the fact that whether a bullet has gone through many viruses or not and making checks for that. This logic obviously did not work as it was actually the viruses that needed to be checked and seen if the bullet passed through them or not. Once I changed my logic and coded accordingly, the function began to work.
Resolution:
- In order to properly implement the bullet mechanics, I decided to nest the bullet class in the player class. This allowed to easily link the data of the player with that of the bullet and made it easy to correlate their positions. Previously, I had experimented with the bullet class inheriting from the player class but realized that the player and bullet do not have a Is-a relationship but have a Has-a relationship and therefore nesting would work best for this problem (I could have used composition but decided to go with nesting).
- The way I tackled the problem of killing the virus with the bullet was by creating a function that would check if the bullet is in the vicinity of the virus and then running it to check for every virus and every bullet.
Ideation:
- My ideation for this task primarily involved the following:
- Game Concept Idea
- Game Play Idea
Game Concept:
- For the concept, as I had mentioned before, I was inclined towards making a game for the gym slots but this idea of a shooting game, dawned upon me when I was siting in a cafe and just being frustrated over the fact that my mask was irritating me.
Game Play:
- As I started building this game, I knew that I would be building a top down game wherein the virus appears to be falling down the screen and the player is positioned in the end of the screen and can only move in the horizontal direction while his bullets travel vertically up and can interact with the virus to kill them. I believe that for the time being the top down approach works well but I would like to experiment with different view points of playing the game. I tried looking at the first person perspective but apparently that required P3D rendering. However, this could be something I could try out for my midterm project.
Notes:
- While this game solves the purpose of this week’s assignment, I am hopeful that I can develop this much better than this and probably turn it into my midterm project. This version is therefore a prototype that will be worked upon as I learn more about processing in the following weeks.
Concepts Learned:
- This project taught me how to nest classes and how to better link one class to the next.
- It taught me to scout for game art and then effectively apply them in my own projects using the PImage, img and images() functions in Processing.
- This project taught me how to work with the ArrayList function.
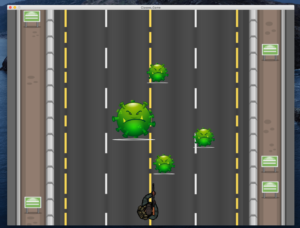
Project Video:
Project Code:
Main draw() Class
ArrayList<Virus> viruses = new ArrayList<Virus>(); //gives the list of viruses Player player; //player object boolean shoot = false; // ensures that the player only shoots when commanded ArrayList<Player.Bullet> bullets = new ArrayList<Player.Bullet>(); //bullet list void setup() { size(1280, 960); imageMode(CENTER); viruses.add(new Virus(random(width*0.25, width*0.75), 0, int(random(1, 3)))); //initialising the list of viruses to spawn in between the roads of the game player = new Player(); } void draw() { PImage bg; bg = loadImage("background-1.png"); bg.resize(int(width),int(height)); background(bg); //background is the top down view of a road Player_Mechanics(); Virus_Mechanics(); } void Player_Mechanics() { player.drawPlayer(); //draws the Player on Screen for(int i =0; i< bullets.size(); i++) { bullets.get(i).draw_Bullet(); bullets.get(i).shoot_Bullet(); //if bullets are present, draws them and shoots them if(bullets.get(i).bullet_posY_1 < 0 ) bullets.remove(i); //removes the bullet once they are out of frame } } void Virus_Mechanics() { for (int i = 0; i < viruses.size(); i++) { viruses.get(i).drawVirus(); viruses.get(i).moveVirus(); //draws and moves virus on screen if(viruses.get(i).posY > height + viruses.get(i).size) viruses.remove(i); //removes the virus once they are out of frame if( bullets.size() > 0 && viruses.size() > 0 ) { for(int j=0; j<bullets.size(); j++) { if( bullets.size() > 0 && viruses.size() > 0 ) { if(viruses.get(i).vicinty_check(bullets.get(j).bullet_posX,bullets.get(j).bullet_posY_2)) { viruses.remove(i); //kills the virus and removes it once bullet touches it } } } } } } void keyPressed() { if (key == ' ') { viruses.add(new Virus(random(width*0.25, width*0.75), 0, int(random(1, 3)))); //spawns a virus } if (key == 'a') { player.moveLeft(); //player goes left } if (key == 'd') { player.moveRight(); //player goes right } if (key == 'f') { bullets.add(player.new Bullet(0)); //shoots bullets } }
Player Class
class Player { public float posX = width/2; public float posY = height-100; PImage player; int movement=20; int size =200; Player() { player = loadImage("player_rifle.png"); imageMode(CENTER); } void drawPlayer() { player.resize(size, 0); pushMatrix(); translate(posX, posY); rotate(radians(270)); image(player, 0, 0); popMatrix(); //resizes and rotates the image of the player on screen so that it is appropriate for the game } void moveLeft() { if (posX > size/2) posX -= movement; //player moves left } void moveRight() { if (posX < width-size/2) posX += movement; //player moves right } class Bullet { //nested bullet class in player class float bullet_posX = posX + size*0.15; float bullet_posY_1 = posY - size/2; float bullet_size = size * 0.1; float bullet_posY_2 = posY - size/2 - bullet_size; int bullet_speed = 20; Bullet(float y) { bullet_posY_1 -= y; bullet_posY_2 -= y; //assigns intial position to bullet } void draw_Bullet() { stroke(195,153,83); strokeWeight(6); line(bullet_posX, bullet_posY_1, bullet_posX, bullet_posY_2); //draws a line for a bullet } void shoot_Bullet() { bullet_posY_1 -= bullet_speed; bullet_posY_2 -= bullet_speed; //moves bullet } } }
Virus Class
class Virus { float posX; float posY; int speed = 2; int size; PImage corona; Virus(float x, float y, int s) { posX = x; posY = y; size = s*100; corona = loadImage("corona.png"); imageMode(CENTER); } void drawVirus() { corona.resize(size, 0); image(corona, posX, posY); //resizes and draws the virus } void moveVirus() { posY += speed; //moves the virus } boolean vicinty_check(float x, float y) { if ((x < posX+size/2 && x > posX-size/2) && (y < posY+size/2 && y > posY-size/2)) //checks if anything exists in the vicintiy of the virus's body return true; else return false; } }