3 hours ago I was sitting in front of my Arduino kit and was absolutely terrified: What on Earth do I do with all these lights and buttons?!!
After a couple of YouTube tutorials and going back to our class recording, I slowly figured out where all these wires go, how to connect a button, and an LED light.
Soo…I managed to get a circuit of 4 buttons and 3 LED lights (let’s call them B1, B2, and G):
- button #0 does not trigger anything,
- button #3 triggers two lights – B1 and G,
- button #2 triggers B1,
- button #1 triggers G.
If you may have noticed, B2 cannot be triggered by pressing on any of the single buttons, so there has to be some trick around this! Your task is to figure out which and trigger all three lights at the same time (preferably with a minimum number of buttons pressed).
The solution is to press button #0 and #1 simultaneously to trigger B2 and G, and then press button #3 to trigger B1.
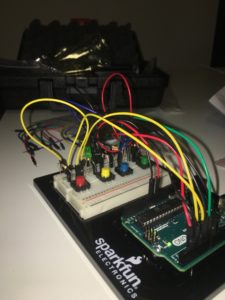
Here is the code:
// declare pins and buttons int ledPin1 = 2; int buttonPin1 = 3; int ledPin2 = 4; int buttonPin2 = 5; int ledPin3 = 7; int buttonPin3 = 6; int buttonPin4 = 8; void setup() { // put your setup code here, to run once: pinMode(ledPin1, OUTPUT); pinMode(buttonPin1, INPUT); Serial.begin(9600); pinMode(ledPin2, OUTPUT); pinMode(buttonPin2, INPUT); Serial.begin(9600); } void loop() { // green light int buttonState1 = digitalRead(buttonPin1); Serial.println(buttonState1); digitalWrite(ledPin2, buttonState1); // HIGH = 1 or true // green light int buttonState2 = digitalRead(buttonPin2); Serial.println(buttonState2); digitalWrite(ledPin2, buttonState2); // blue light int buttonState3 = digitalRead(buttonPin3); Serial.println(buttonState3); digitalWrite(ledPin3, buttonState3); // blue and green int buttonState4 = digitalRead(buttonPin4); if (buttonState4 == buttonState1) digitalWrite(ledPin1, buttonState4); }