I struggled at first to actually start doing anything that is meaningful because I like to plan things ahead and foreseeing how the coding will go is quite difficult. I took inspiration from my Snapchat Bitmoji, knowing it’s just for ideas not copying.
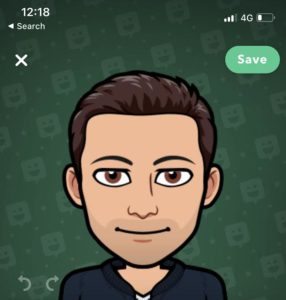
I made some quick research on Processing’s Reference pages and decided that the beginShape() might be a very suitable method for drawing the face, hair, and eyes (basically figures I thought would require complex drawing to be perfected).
I tried out the function and this is what I was able to do before realizing that the shape of the face can just be an ellipse for the sake of simplicity and because beginshape() will take a very long time to draw a curved shape.
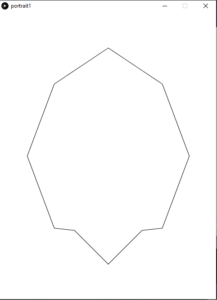
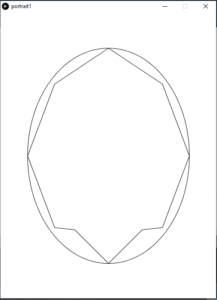
I then started to master the small operations that I code for each part of the portrait, and I drew a very detailed eye which contains the eye itself, the brown iris, the white sclera, the pupil, and dark brown eyebrows.
Even though the eye was on point, I realized that making the portrait realistic will just make it weird and ugly so the rest of the face should just be more cartoon-like.
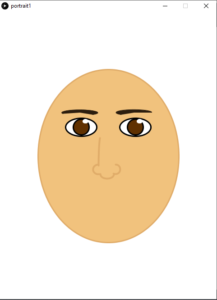
I drew a simple nose with just one outline from the right and some detail at the bottom, and I made a very simplistic but appropriate slightly-smiling mouth. The main function used was the “arc” function and I also struggled at first to understand how the radian angles calculations were carried out but I got things right simply by very long trial and error.
After completing most of the face, I decided to draw the hair using the beginShape() function which I struggled with earlier, and it turned it to be extremely useful for irregular shapes. By now, I was almost blindly typing numbers and automatically making calculations of dimensions and distances.
I made the ears using another arc function, and I decided to make the background a still photo of something that represents me; squash. I played squash for 5 years and even though I stopped playing years ago, I adore the sport and still play for fun until today.
Lastly, I decided to make use of the clicking of mouse function in a simple creative way where if you click the mouse the colors of the eyes and the clothing randomly change simultaneously.
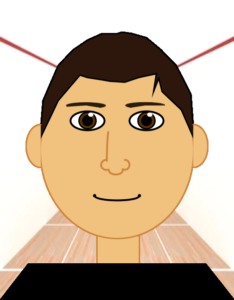
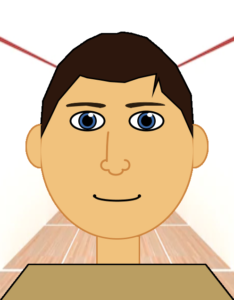
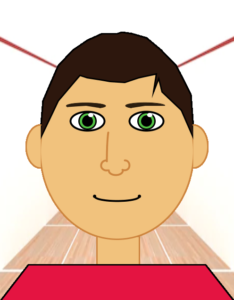
//Assignment 1 Yahia Beethoven Tayel self portrait //Initializing the variables before any function //In case the image doesn't load int backgroundColor = 255; //dimesnions of the portrait float x; float y; //randomized variables for the color of the clothes when mouseclicked float r = 0; float g = 0; float b = 0; float r1 = 96; float g1 = 49; float b1 = 1; //setting up the plane on which the portrait is drawn void setup() { size(500, 640); x = width/2; y = height/2; PImage img; img = loadImage("squash_background.PNG"); img.resize(500, 640); background(backgroundColor); background(img); } //the main draw function that makes the face void draw() { //draws neck fill(241, 194, 125); stroke(205, 133, 63); rect(x - 43, y+180, 90, 60); //draws the face strokeWeight(3); fill(241, 194, 125); stroke(205, 133, 63); ellipse(x, y, 6.5*x/5, 7*y/6); //draws the hair strokeWeight(3); fill(45, 23, 13); stroke(0); beginShape(); vertex(x/4 + 24, y - 25); vertex(3*x/8, 2*y/3); vertex(x/2, y/2.3); vertex(5*x/8, y/3); vertex(3*x/4, y/3.75); vertex(7*x/8, y/4.45); vertex(x, y/4.5); vertex(x+10, y/4.7); vertex(9*x/8, y/4.45); vertex(5*x/4, y/4); vertex(11*x/8, y/3); vertex(3*x/2, y/2.3); vertex(13*x/8, 2*y/3.2); vertex(x+3*x/4 - 24, y - 25); vertex(13*x/8 - 20, 2*y/3 +20); vertex(3*x/2 - 30, y/2.3 +55); vertex(11*x/8 - 5, y/3 +60); vertex(5*x/4 +15, y/2.3 +55); vertex(11*x/8 - 10, y/3 +50); vertex(9*x/8 +15, y/2.3 +25); vertex(9*x/8 - 15, y/2.3 +35); vertex(x- 15, y/2.3 +33); vertex(7*x/8, y/2.3 +30); vertex(5*x/8 +30, y/2.3 +25); vertex(5*x/8 +15, y/2.3 +20); vertex(x/2, 2*y/3); endShape(CLOSE); //draws the eyes strokeWeight(4); fill(255); stroke(0); ellipse(3*x/4, 4*y/5, x/3.5, y/8); ellipse(5*x/4, 4*y/5, x/3.5, y/8); //draws the iris strokeWeight(3); fill(r1, g1, b1); stroke(0); ellipse(3*x/4, 4*y/5, x/7, y/9); ellipse(5*x/4, 4*y/5, x/7, y/9); //draws the pupil fill(0); stroke(0); ellipse(3*x/4, 4*y/5, x/13, y/15); ellipse(5*x/4, 4*y/5, x/13, y/15); //draws the white spot strokeWeight(1); fill(255); stroke(255); ellipse(3*x/4 + x/38, 4*y/5 - y/25, x/25, y/40); ellipse(5*x/4 + x/38, 4*y/5 - y/25, x/25, y/40); //draws the eyebrows strokeWeight(1); fill(43, 29, 14); stroke(43, 29, 14); arc(3*x/4 - 3, 4*y/5-y/10, x/3, 13, HALF_PI+QUARTER_PI + 0.4, TWO_PI+QUARTER_PI/2 +0.4); arc(5*x/4 -3 , 4*y/5-y/10, x/3, 13, HALF_PI+QUARTER_PI, TWO_PI+QUARTER_PI/2 - 0.4); //draws the nose noFill(); stroke(205, 133, 63); strokeWeight(4); //arc(x+12, 5*y/6 + 10, 7, y/2.5, 0, HALF_PI); arc(x-18, 5*y/6 + 75, 7, y/2.5, PI, PI+QUARTER_PI+0.5); //took the two nostrils idea from aysha of this class arc(x - 23, y+30, 21, 21, HALF_PI-QUARTER_PI/3, PI+2*QUARTER_PI); arc(x+16, y+30, 21, 21, -HALF_PI, HALF_PI+QUARTER_PI/2); arc(x-3, y+40, 30, 21, 0, PI); //draws the mouth noFill(); stroke(0); arc(x, y+95, 95, 21, 0, PI); strokeWeight(7); point(x+47.5, y+95); point(x-47.5, y+95); //draws the ears strokeWeight(3); fill(241, 194, 125); stroke(205, 133, 63); arc(x/4 + 26, y - 10, x/4, y/3.5, HALF_PI, PI+HALF_PI ); arc(x+3*x/4 - 26, y - 10, x/4, y/3.5, -HALF_PI, PI-HALF_PI); //draws shoulders fill(r, g, b); stroke(0); beginShape(); vertex(0, 2*y); vertex(x/4, y+240); vertex(x+3*x/4, y+240); vertex(2*x, 2*y); endShape(); } //functions that are called when the mouse is clicked to randomly change the colors of both the eyes and the clothes simultaneously void mousePressed() { r = random(255); g = random(255); b = random(255); r1 = random(255); g1 = random(255); b1 = random(255); }
I learned a lot through this simple assignment and I look forward to future assignments which will help develop my skills in programming much more.