Disclaimer: things in bold are little nuggets of knowledge that might come in useful. And notes to self, so I don’t make dumb mistakes again.
I love videogames. And colorful blinking lights, obviously. Given that I can’t make a full-blown videogame just yet, I decided to start with something simpler (but challenging given that this is just my second project). The goal: build and program a memory game (Simon).
Step 1 was making the LEDs actually turn on. Four buttons acted as inputs, and the LEDs were connected as four outputs. I could have as well made the LEDs turn on via the physical switches, but that would have defeated the purpose of the asignment. Nothing too different from what we did in class. It was simple enough to code, but it didn’t work out at the first go. My brain forgot to plug the outputs to ground (way to go – brain-five!). You can see the result here: https://youtu.be/yzMvNNp7sp4
And then the fun part started.

Step 2: get a simplified version running. By that I mean getting the device to flash one light, waiting for a player input, and then telling them if they were right or wrong. First, the system would pull a random number between 0 and 3 and store it in a variable. Each button/color would correspond to a number in base 4 (I swear it’s not as complicated as it sounds). The device would flash the corresponding color, and then the player would make an input. Comparing the input and the stored number, the game would decide if it was right or wrong.
The hardest part of this was getting randomization to work. You see, to get the function random() to actually work, one has first to create a seed using random noise from an unplugged pin 0 and the function randomSeed(). Not too hard to do once you read a bit of the reference manual. The problem is that if I do that inside void setup(), it runs once (as intended) but then the variable cannot be retrieved from within void loop(). But if I generate the number within the loop, it would keep creating numbers forever.
To solve this, I created a global variable (don’t forget the semicolon or the whole thing dies doesn’t compile!) that allowed the program to count the loop repetitions, in a way. This way, the randomization would run only once within the loop, and then the program would just compare the input. Add an RGB LED and you get a purple light for victory!
The result is here: https://youtu.be/LhxNZv7ddL8
Step 3: find a way to add another light to the sequence. By recursion/induction/all things math, if this worked, I would be able to add as many lights as I wanted (though my method is probably not the most efficient). This time, I generated random numbers from 0 to 15. That way, the sequence of two lights would represent a two-digit number in base 4. The first and second player inputs would be stored in different variables, one representing 4s and other representing 1s. For instance, the number 13 (base 10) would be 31 in base 4, or a blue-yellow sequence. To make the initial flashing sequence work, I just had to get the first light to represent 4s by dividing the random number by 4 (int divisions return only the intger part – no rounding!) and the second to represent 1s by using the modulo (%) operator.
To keep the inputs separate, I assigned the input variables the value 4 as a placeholder, such that the program could determine if the variables had values different than 4 (i.e. the player had made an input) and enter different conditionals based on that. In the end, I combine both variables into a single number again and compare it with the random number. Purple light for yay, red light for boo.
After that, adding a third light to the sequence was trivial. The final game is here: https://youtu.be/lQSuhgRGnn8
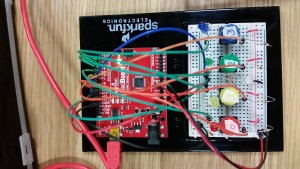
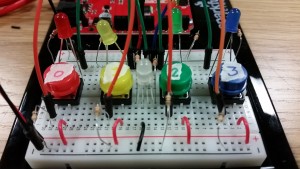
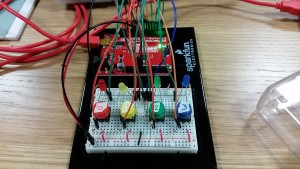
Step 4 (BONUS): I had another idea for a slighlty different game with a similar program. The program generates a random number from 0 to 63 which in base 4 represents a 3-button password. After every attempt at guessing the password, the player is provided with feedback: blue light if his number is lower than the password, red if it’s higher, and purple if the player is right. So yeah, it’s just about guessing and using the light to get closer to the answer. Yay. You can watch it here: https://youtu.be/8-ZTPJAOZ5w